C# 이벤트와 델리게이트 이해하기 🚀
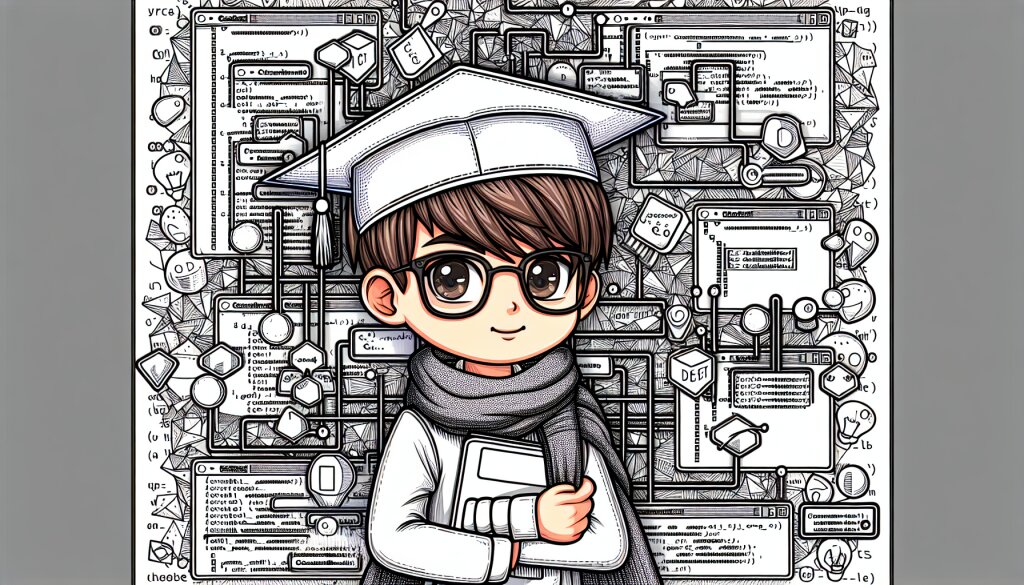
1. 들어가며: C#의 강력한 기능, 이벤트와 델리게이트 🎭
안녕하세요, 프로그래밍 열정가 여러분! 오늘은 C#의 핵심 기능 중 두 가지인 이벤트(Event)와 델리게이트(Delegate)에 대해 깊이 있게 알아보려고 합니다. 이 두 개념은 C# 프로그래밍에서 매우 중요한 역할을 하며, 특히 객체 지향 프로그래밍과 이벤트 기반 프로그래밍에서 핵심적인 요소입니다.
이벤트와 델리게이트는 마치 재능넷(https://www.jaenung.net)에서 다양한 재능을 연결하듯, 프로그램 내의 다양한 부분을 유연하게 연결해주는 역할을 합니다. 재능넷이 재능 공유의 플랫폼이라면, 이벤트와 델리게이트는 코드 내에서 기능을 공유하고 연결하는 플랫폼이라고 볼 수 있죠. 😊
이 글을 통해 여러분은 다음과 같은 내용을 배우게 될 것입니다:
- ✅ 델리게이트의 개념과 사용법
- ✅ 이벤트의 정의와 작동 원리
- ✅ 델리게이트와 이벤트의 차이점
- ✅ 실제 프로그래밍에서의 활용 사례
- ✅ 고급 기법과 최적화 방법
자, 그럼 C#의 매력적인 세계로 함께 빠져볼까요? 🎈
2. 델리게이트(Delegate)의 기초 🧱
델리게이트는 C#에서 매우 중요한 개념 중 하나입니다. 간단히 말해, 델리게이트는 메서드를 참조하는 타입입니다. 이는 마치 객체가 데이터를 담는 것처럼, 델리게이트는 메서드를 담는 그릇이라고 생각하면 됩니다.
2.1 델리게이트의 정의
델리게이트는 다음과 같은 형식으로 정의됩니다:
delegate 반환타입 델리게이트이름(매개변수들);
예를 들어, 정수 두 개를 받아 정수를 반환하는 메서드를 참조하는 델리게이트는 다음과 같이 정의할 수 있습니다:
delegate int MathOperation(int x, int y);
2.2 델리게이트의 사용
델리게이트를 사용하는 방법은 다음과 같습니다:
public static int Add(int x, int y)
{
return x + y;
}
public static void Main()
{
MathOperation operation = Add;
int result = operation(5, 3);
Console.WriteLine(result); // 출력: 8
}
여기서 operation
은 Add
메서드를 참조하고 있습니다. 이렇게 하면 operation(5, 3)
은 실제로 Add(5, 3)
을 호출하는 것과 같습니다.
2.3 델리게이트의 장점
델리게이트의 주요 장점은 다음과 같습니다:
- 유연성: 런타임에 메서드를 동적으로 선택하고 호출할 수 있습니다.
- 콜백 메커니즘: 다른 메서드에 메서드를 인자로 전달할 수 있습니다.
- 이벤트 처리: 이벤트 기반 프로그래밍의 기초가 됩니다.
2.4 멀티캐스트 델리게이트
C#의 델리게이트는 여러 메서드를 동시에 참조할 수 있는 '멀티캐스트' 기능을 제공합니다. 이는 +=
연산자를 사용하여 구현할 수 있습니다:
public static void Hello() { Console.WriteLine("Hello"); }
public static void World() { Console.WriteLine("World"); }
public static void Main()
{
Action multiDelegate = Hello;
multiDelegate += World;
multiDelegate(); // 출력: Hello\nWorld
}
이 예제에서 multiDelegate
를 호출하면 Hello
와 World
메서드가 순차적으로 실행됩니다.
2.5 제네릭 델리게이트
C#은 Func<>
와 Action<>
과 같은 제네릭 델리게이트를 제공합니다. 이들을 사용하면 많은 경우에 사용자 정의 델리게이트를 만들 필요 없이 바로 사용할 수 있습니다.
Func<int, int, int> add = (x, y) => x + y;
int result = add(5, 3); // result: 8
Action<string> greet = name => Console.WriteLine($"Hello, {name}!");
greet("Alice"); // 출력: Hello, Alice!
Func<>
는 반환 값이 있는 메서드를, Action<>
은 반환 값이 없는 메서드를 나타냅니다.
3. 이벤트(Event)의 이해 🎉
이벤트는 객체의 상태 변화나 특정 동작의 발생을 다른 객체에게 알리는 메커니즘입니다. C#에서 이벤트는 델리게이트를 기반으로 구현되며, 발행-구독(publish-subscribe) 모델을 따릅니다.
3.1 이벤트의 정의
이벤트는 다음과 같이 정의됩니다:
public event EventHandler SomeEvent;
여기서 EventHandler
는 .NET에서 제공하는 기본 이벤트 델리게이트 타입입니다.
3.2 이벤트의 사용
이벤트를 사용하는 기본적인 패턴은 다음과 같습니다:
public class Button
{
public event EventHandler Click;
protected virtual void OnClick(EventArgs e)
{
Click?.Invoke(this, e);
}
public void PerformClick()
{
OnClick(EventArgs.Empty);
}
}
public class Program
{
static void Main()
{
Button button = new Button();
button.Click += Button_Click;
button.PerformClick();
}
static void Button_Click(object sender, EventArgs e)
{
Console.WriteLine("Button was clicked!");
}
}
이 예제에서 Button
클래스는 Click
이벤트를 정의하고, Program
클래스에서 이 이벤트에 대한 핸들러를 등록합니다.
3.3 이벤트의 장점
이벤트를 사용하면 다음과 같은 이점이 있습니다:
- 느슨한 결합: 이벤트 발생 객체와 처리 객체 간의 의존성을 줄입니다.
- 확장성: 새로운 구독자를 쉽게 추가할 수 있습니다.
- 캡슐화: 이벤트 발생 로직을 숨길 수 있습니다.
3.4 사용자 정의 이벤트 인자
때로는 이벤트와 함께 추가 정보를 전달해야 할 때가 있습니다. 이를 위해 사용자 정의 이벤트 인자를 만들 수 있습니다:
public class CustomEventArgs : EventArgs
{
public string Message { get; set; }
}
public class Publisher
{
public event EventHandler<CustomEventArgs> CustomEvent;
protected virtual void OnCustomEvent(CustomEventArgs e)
{
CustomEvent?.Invoke(this, e);
}
public void RaiseEvent()
{
OnCustomEvent(new CustomEventArgs { Message = "Hello, Event!" });
}
}
public class Subscriber
{
public void HandleCustomEvent(object sender, CustomEventArgs e)
{
Console.WriteLine($"Received: {e.Message}");
}
}
public class Program
{
static void Main()
{
Publisher publisher = new Publisher();
Subscriber subscriber = new Subscriber();
publisher.CustomEvent += subscriber.HandleCustomEvent;
publisher.RaiseEvent(); // 출력: Received: Hello, Event!
}
}
이 예제에서는 CustomEventArgs
를 정의하여 이벤트와 함께 추가 정보(Message
)를 전달합니다.
3.5 이벤트와 델리게이트의 차이점
이벤트와 델리게이트는 밀접한 관련이 있지만, 몇 가지 중요한 차이점이 있습니다:
- 접근성: 이벤트는 외부에서 직접 호출할 수 없으며, 오직 정의된 클래스 내에서만 발생시킬 수 있습니다.
- 구독 관리: 이벤트는
+=
와-=
연산자만을 통해 구독자를 추가하거나 제거할 수 있습니다. - Null 체크: 이벤트는 자동으로 null 체크를 수행하여 NullReferenceException을 방지합니다.
이러한 차이점들로 인해 이벤트는 델리게이트보다 더 안전하고 캡슐화된 방식으로 사용될 수 있습니다.
4. 실제 프로그래밍에서의 활용 사례 💼
이벤트와 델리게이트는 실제 프로그래밍에서 다양한 방식으로 활용됩니다. 여기서는 몇 가지 주요 사용 사례를 살펴보겠습니다.
4.1 GUI 프로그래밍
GUI(그래픽 사용자 인터페이스) 프로그래밍에서 이벤트는 매우 중요한 역할을 합니다. 버튼 클릭, 마우스 이동, 키보드 입력 등 사용자의 모든 동작이 이벤트로 처리됩니다.
using System.Windows.Forms;
public class MyForm : Form
{
private Button myButton;
public MyForm()
{
myButton = new Button();
myButton.Text = "Click me!";
myButton.Click += MyButton_Click;
this.Controls.Add(myButton);
}
private void MyButton_Click(object sender, EventArgs e)
{
MessageBox.Show("Button was clicked!");
}
}
이 예제에서 MyButton_Click
메서드는 버튼의 Click
이벤트에 대한 이벤트 핸들러입니다.
4.2 비동기 프로그래밍
델리게이트와 이벤트는 비동기 프로그래밍에서도 유용하게 사용됩니다. 긴 작업이 완료되었을 때 알림을 받는 데 사용할 수 있습니다.
public class AsyncOperation
{
public event EventHandler<OperationCompletedEventArgs> OperationCompleted;
public async Task PerformLongOperation()
{
await Task.Delay(5000); // 5초 동안 작업 수행을 시뮬레이션
OnOperationCompleted(new OperationCompletedEventArgs { Result = "Operation completed successfully" });
}
protected virtual void OnOperationCompleted(OperationCompletedEventArgs e)
{
OperationCompleted?.Invoke(this, e);
}
}
public class OperationCompletedEventArgs : EventArgs
{
public string Result { get; set; }
}
// 사용 예:
AsyncOperation operation = new AsyncOperation();
operation.OperationCompleted += (sender, e) => Console.WriteLine(e.Result);
await operation.PerformLongOperation();
이 예제에서 PerformLongOperation
메서드는 비동기적으로 실행되며, 작업이 완료되면 OperationCompleted
이벤트를 발생시킵니다.
4.3 옵저버 패턴
이벤트는 옵저버 패턴을 구현하는 데 이상적입니다. 이 패턴은 객체의 상태 변화를 다른 객체들에게 자동으로 알리는 데 사용됩니다.
public class WeatherStation
{
private float temperature;
public event EventHandler<TemperatureChangedEventArgs> TemperatureChanged;
public float Temperature
{
get { return temperature; }
set
{
if (temperature != value)
{
temperature = value;
OnTemperatureChanged(new TemperatureChangedEventArgs(value));
}
}
}
protected virtual void OnTemperatureChanged(TemperatureChangedEventArgs e)
{
TemperatureChanged?.Invoke(this, e);
}
}
public class TemperatureChangedEventArgs : EventArgs
{
public float NewTemperature { get; }
public TemperatureChangedEventArgs(float newTemperature)
{
NewTemperature = newTemperature;
}
}
public class TemperatureDisplay
{
public void Subscribe(WeatherStation station)
{
station.TemperatureChanged += HandleTemperatureChanged;
}
private void HandleTemperatureChanged(object sender, TemperatureChangedEventArgs e)
{
Console.WriteLine($"Temperature changed to {e.NewTemperature}°C");
}
}
// 사용 예:
WeatherStation station = new WeatherStation();
TemperatureDisplay display = new TemperatureDisplay();
display.Subscribe(station);
station.Temperature = 25.5f; // 출력: Temperature changed to 25.5°C
station.Temperature = 26.0f; // 출력: Temperature changed to 26°C
이 예제에서 WeatherStation
은 온도 변화를 감지하고 이를 구독자들(TemperatureDisplay
)에게 알립니다.
4.4 플러그인 아키텍처
델리게이트는 플러그인 아키텍처를 구현하는 데 유용합니다. 이를 통해 프로그램의 기능을 동적으로 확장할 수 있습니다.
public interface IPlugin
{
string Name { get; }
void Execute();
}
public class PluginManager
{
private Dictionary<string, Func<IPlugin>> plugins = new Dictionary<string, Func<IPlugin>>();
public void RegisterPlugin(string name, Func<IPlugin> creator)
{
plugins[name] = creator;
}
public void ExecutePlugin(string name)
{
if (plugins.TryGetValue(name, out var creator))
{
IPlugin plugin = creator();
plugin.Execute();
}
else
{
Console.WriteLine($"Plugin '{name}' not found.");
}
}
}
// 플러그인 예:
public class HelloPlugin : IPlugin
{
public string Name => "Hello";
public void Execute()
{
Console.WriteLine("Hello from plugin!");
}
}
// 사용 예:
PluginManager manager = new PluginManager();
manager.RegisterPlugin("Hello", () => new HelloPlugin());
manager.ExecutePlugin("Hello"); // 출력: Hello from plugin!
이 예제에서 PluginManager
는 델리게이트(Func<IPlugin>
)를 사용하여 플러그인을 동적으로 생성하고 실행합니다.
4.5 콜백 메커니즘
델리게이트는 콜백 메커니즘을 구현하는 데 자주 사용됩니다. 이는 특히 비동기 작업이나 이벤트 처리에 유용합니다.
public class DataFetcher
{
public void FetchData(string url, Action<string> onSuccess, Action<Exception> onError)
{
try
{
// 데이터를 가져오는 작업을 시뮬레이션
string data = $"Data from {url}";
onSuccess(data);
}
catch (Exception ex)
{
onError(ex);
}
}
}
// 사용 예:
DataFetcher fetcher = new DataFetcher();
fetcher.FetchData("https://example.com",
data => Console.WriteLine($"Received: {data}"),
error => Console.WriteLine($"Error: {error.Message}"));
이 예제에서 FetchData
메서드는 두 개의 콜백(성공 시와 실패 시)을 매개변수로 받아 적절한 상황에 호출합니다.
5. 고급 기법과 최적화 방법 🚀
이벤트와 델리게이트를 효과적으로 사용하기 위해서는 몇 가지 고급 기법과 최적화 방법을 알아두는 것이 좋습니다. 이 섹션에서는 이러한 고급 주제들을 다루겠습니다.
5.1 약한 이벤트 패턴
메모리 누수를 방지하기 위해 약한 이벤트 패턴을 사용할 수 있습니다. 이는 이벤트 발행자가 구독자에 대한 강한 참조를 유지하지 않도록 합니다.