C에서의 객체 지향 프로그래밍 모방 🚀
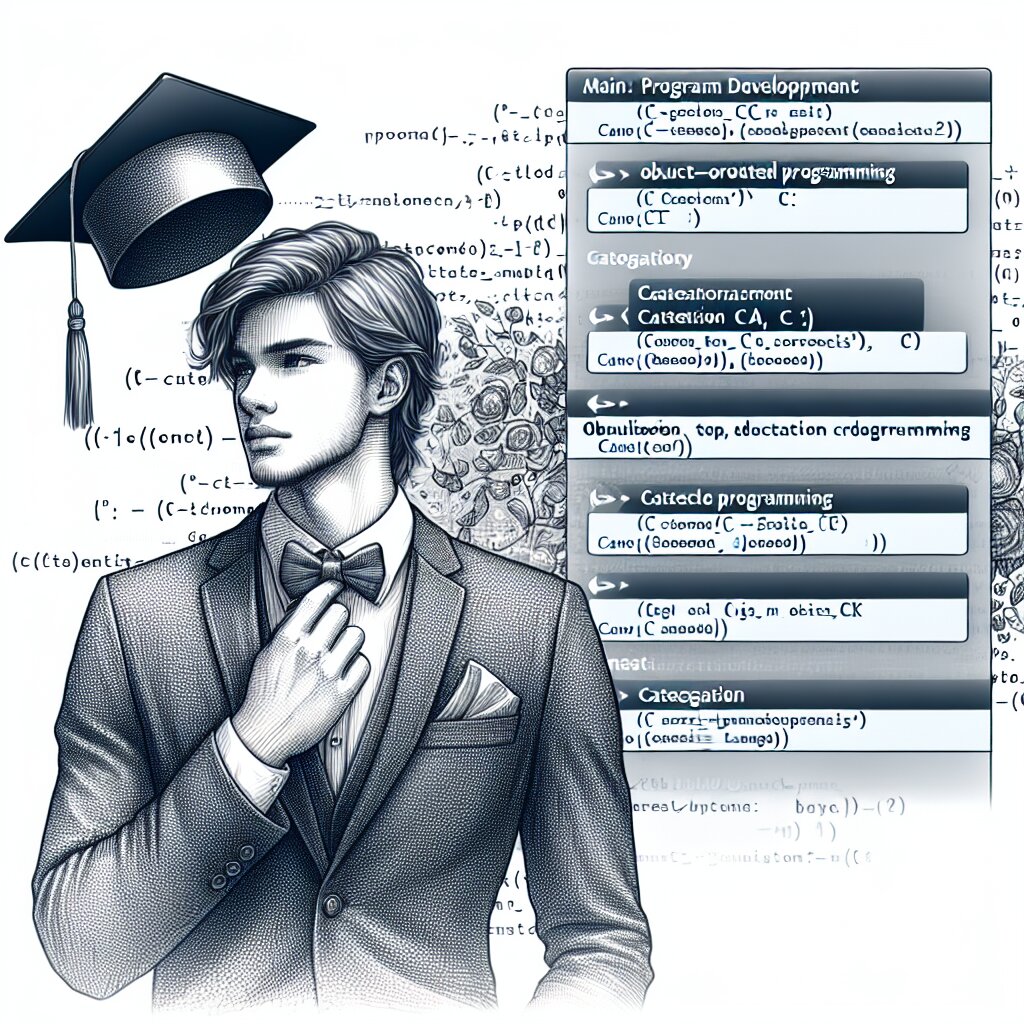
안녕, 친구들! 오늘은 정말 재미있는 주제로 이야기를 나눠볼 거야. 바로 C 언어에서 객체 지향 프로그래밍을 어떻게 흉내 낼 수 있는지에 대해서 말이야. 😎 C는 절차적 언어로 알려져 있지만, 우리가 조금만 창의적으로 생각하면 객체 지향의 맛을 낼 수 있다고? 믿기 힘들겠지만 진짜야!
이 여정을 통해 우리는 C의 숨겨진 매력을 발견하고, 프로그래밍의 새로운 차원을 경험하게 될 거야. 마치 재능넷에서 새로운 재능을 발견하는 것처럼 말이지! 자, 그럼 이제 C로 객체 지향의 세계로 뛰어들어볼까?
1. 객체 지향 프로그래밍이란? 🤔
먼저, 객체 지향 프로그래밍(OOP)이 뭔지 간단히 알아보자. OOP는 프로그램을 객체들의 모음으로 디자인하는 프로그래밍 패러다임이야. 각 객체는 데이터(속성)와 코드(메서드)를 가지고 있지. 이게 왜 좋냐고? 코드를 더 구조화하고, 재사용성을 높이고, 유지보수를 쉽게 만들어주거든!
OOP의 주요 특징:
- 캡슐화 (Encapsulation)
- 상속 (Inheritance)
- 다형성 (Polymorphism)
- 추상화 (Abstraction)
이제 우리의 미션은 이런 특징들을 C에서 어떻게 구현할 수 있을지 알아보는 거야. 마치 재능넷에서 새로운 기술을 배우는 것처럼 흥미진진하겠지? 😄
2. C에서 '객체' 만들기 🏗️
자, 이제 본격적으로 C에서 '객체'를 만들어보자. C에는 클래스라는 개념이 없지만, 구조체(struct)를 이용해서 비슷한 걸 만들 수 있어.
예를 들어, 강아지 객체를 만든다고 생각해보자:
typedef struct {
char name[50];
int age;
char breed[50];
} Dog;
이렇게 하면 강아지의 이름, 나이, 품종을 가진 '객체'가 만들어졌어! 근데 잠깐, 객체는 데이터뿐만 아니라 행동(메서드)도 가져야 하잖아? C에서는 이걸 어떻게 구현할 수 있을까?
바로 함수 포인터를 사용하는 거야! 😎
typedef struct {
char name[50];
int age;
char breed[50];
void (*bark)(void); // 함수 포인터
} Dog;
void dogBark() {
printf("Woof! Woof!\n");
}
// 사용 예
Dog myDog = {"Buddy", 3, "Labrador", dogBark};
myDog.bark(); // "Woof! Woof!" 출력
와우! 이제 우리의 강아지 '객체'는 데이터도 가지고 있고, 짖을 수도 있어! 🐶
🌟 Pro Tip: 함수 포인터를 사용하면 다형성도 어느 정도 구현할 수 있어. 예를 들어, 다른 품종의 강아지는 다르게 짖게 만들 수 있지!
이렇게 C에서도 객체와 비슷한 걸 만들 수 있다니, 신기하지 않아? 마치 재능넷에서 새로운 재능을 발견한 것 같은 기분이야! 🎉
3. 캡슐화: 데이터를 숨기자! 🙈
객체 지향 프로그래밍의 중요한 특징 중 하나가 바로 캡슐화야. 캡슐화는 객체의 내부 데이터를 외부에서 직접 접근하지 못하게 하고, 대신 메서드를 통해 접근하게 하는 거야. 이렇게 하면 데이터의 무결성을 지킬 수 있지.
C에서는 어떻게 이걸 구현할 수 있을까? 여기 재미있는 트릭이 있어!
// Dog.h
typedef struct Dog Dog;
Dog* createDog(const char* name, int age, const char* breed);
void destroyDog(Dog* dog);
void dogBark(Dog* dog);
const char* getDogName(Dog* dog);
void setDogAge(Dog* dog, int age);
// Dog.c
#include "Dog.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Dog {
char name[50];
int age;
char breed[50];
};
Dog* createDog(const char* name, int age, const char* breed) {
Dog* dog = malloc(sizeof(Dog));
strncpy(dog->name, name, 49);
dog->age = age;
strncpy(dog->breed, breed, 49);
return dog;
}
void destroyDog(Dog* dog) {
free(dog);
}
void dogBark(Dog* dog) {
printf("%s says: Woof! Woof!\n", dog->name);
}
const char* getDogName(Dog* dog) {
return dog->name;
}
void setDogAge(Dog* dog, int age) {
if (age > 0 && age < 20) { // 유효성 검사
dog->age = age;
}
}
</stdio.h></string.h></stdlib.h>
이렇게 하면 Dog 구조체의 실제 정의는 .c 파일에 숨겨져 있고, 외부에서는 Dog 포인터를 통해서만 접근할 수 있어. 구조체의 멤버에 직접 접근할 수 없으니까, getter와 setter 함수를 통해서만 데이터를 읽고 쓸 수 있지.
💡 Insight: 이 방식은 C에서 정보 은닉을 구현하는 고전적인 방법이야. 라이브러리를 만들 때 자주 사용되는 테크닉이지!
사용 예:
// main.c
#include "Dog.h"
#include <stdio.h>
int main() {
Dog* myDog = createDog("Buddy", 3, "Labrador");
dogBark(myDog);
printf("%s is %d years old.\n", getDogName(myDog), getDogAge(myDog));
setDogAge(myDog, 4);
destroyDog(myDog);
return 0;
}
</stdio.h>
이렇게 하면 Dog의 내부 구현은 완전히 숨겨지고, 사용자는 제공된 함수들을 통해서만 Dog와 상호작용할 수 있어. 멋지지 않아? 😎
이런 식으로 C에서도 캡슐화를 구현할 수 있다니, 정말 신기하지 않아? 마치 재능넷에서 숨겨진 재능을 발견한 것 같은 기분이야! 🎭
4. 상속: 기능을 물려받자! 👨👦
객체 지향 프로그래밍의 또 다른 중요한 특징은 바로 상속이야. 상속을 통해 기존의 클래스(부모 클래스)로부터 속성과 메서드를 물려받아 새로운 클래스(자식 클래스)를 만들 수 있지. 이렇게 하면 코드 재사용성이 높아지고, 계층 구조를 만들 수 있어.
C에는 클래스가 없으니까 상속도 없겠지? 라고 생각했다면 오산이야! C에서도 구조체를 이용해서 상속과 비슷한 효과를 낼 수 있어. 어떻게 하는지 한번 볼까?
// Animal.h
typedef struct Animal Animal;
Animal* createAnimal(const char* name, int age);
void destroyAnimal(Animal* animal);
void animalSpeak(Animal* animal);
const char* getAnimalName(Animal* animal);
int getAnimalAge(Animal* animal);
// Animal.c
#include "Animal.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Animal {
char name[50];
int age;
};
Animal* createAnimal(const char* name, int age) {
Animal* animal = malloc(sizeof(Animal));
strncpy(animal->name, name, 49);
animal->age = age;
return animal;
}
void destroyAnimal(Animal* animal) {
free(animal);
}
void animalSpeak(Animal* animal) {
printf("%s makes a sound.\n", animal->name);
}
const char* getAnimalName(Animal* animal) {
return animal->name;
}
int getAnimalAge(Animal* animal) {
return animal->age;
}
// Dog.h
typedef struct Dog Dog;
Dog* createDog(const char* name, int age, const char* breed);
void destroyDog(Dog* dog);
void dogBark(Dog* dog);
const char* getDogBreed(Dog* dog);
// Dog.c
#include "Dog.h"
#include "Animal.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Dog {
Animal base; // '상속'
char breed[50];
};
Dog* createDog(const char* name, int age, const char* breed) {
Dog* dog = malloc(sizeof(Dog));
Animal* base = (Animal*)dog;
*base = *createAnimal(name, age);
strncpy(dog->breed, breed, 49);
return dog;
}
void destroyDog(Dog* dog) {
destroyAnimal((Animal*)dog);
}
void dogBark(Dog* dog) {
printf("%s (a %s) says: Woof! Woof!\n", getAnimalName((Animal*)dog), dog->breed);
}
const char* getDogBreed(Dog* dog) {
return dog->breed;
}
</stdio.h></string.h></stdlib.h></stdio.h></string.h></stdlib.h>
여기서 우리는 Animal을 '부모 클래스'로, Dog를 '자식 클래스'로 만들었어. Dog 구조체의 첫 번째 멤버로 Animal 구조체를 넣음으로써 '상속'을 구현한 거지. 이렇게 하면 Dog는 Animal의 모든 속성을 가지면서 추가적인 속성(breed)도 가질 수 있어.
🚀 Advanced Tip: 이 방식을 "구조체 내포(struct embedding)"라고 불러. C++의 상속 구현 방식과 비슷하지만, 가상 함수 테이블 같은 건 직접 구현해야 해.
사용 예를 한번 볼까?
// main.c
#include "Animal.h"
#include "Dog.h"
#include <stdio.h>
int main() {
Animal* genericAnimal = createAnimal("Generic", 5);
Dog* myDog = createDog("Buddy", 3, "Labrador");
animalSpeak(genericAnimal); // "Generic makes a sound."
animalSpeak((Animal*)myDog); // "Buddy makes a sound."
dogBark(myDog); // "Buddy (a Labrador) says: Woof! Woof!"
printf("%s is %d years old and is a %s.\n",
getAnimalName((Animal*)myDog),
getAnimalAge((Animal*)myDog),
getDogBreed(myDog));
destroyAnimal(genericAnimal);
destroyDog(myDog);
return 0;
}
</stdio.h>
이렇게 하면 Dog 객체를 Animal 포인터로 캐스팅해서 사용할 수도 있고, Dog만의 고유한 메서드(dogBark)도 사용할 수 있어. 마치 진짜 상속처럼 동작하는 거지!
C에서 이렇게 상속을 구현할 수 있다니, 정말 놀랍지 않아? 마치 재능넷에서 숨겨진 재능을 발견한 것 같은 기분이야! 🎭 이제 우리는 C에서도 복잡한 객체 계층 구조를 만들 수 있게 됐어!
5. 다형성: 같은 이름, 다른 행동! 🎭
다형성은 객체 지향 프로그래밍의 꽃이라고 할 수 있어. 같은 인터페이스를 사용하지만 객체에 따라 다르게 동작하는 거지. C++나 Java에서는 가상 함수나 인터페이스를 통해 이를 쉽게 구현할 수 있어. 그럼 C에서는 어떻게 할 수 있을까? 😏
C에서 다형성을 구현하는 방법 중 하나는 함수 포인터를 사용하는 거야. 이전에 만든 Animal과 Dog 예제를 조금 수정해서 다형성을 구현해보자!
// Animal.h
typedef struct Animal Animal;
Animal* createAnimal(const char* name, int age, void (*speak)(Animal*));
void destroyAnimal(Animal* animal);
void animalSpeak(Animal* animal);
const char* getAnimalName(Animal* animal);
int getAnimalAge(Animal* animal);
// Animal.c
#include "Animal.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Animal {
char name[50];
int age;
void (*speak)(Animal*);
};
Animal* createAnimal(const char* name, int age, void (*speak)(Animal*)) {
Animal* animal = malloc(sizeof(Animal));
strncpy(animal->name, name, 49);
animal->age = age;
animal->speak = speak;
return animal;
}
void destroyAnimal(Animal* animal) {
free(animal);
}
void animalSpeak(Animal* animal) {
animal->speak(animal);
}
const char* getAnimalName(Animal* animal) {
return animal->name;
}
int getAnimalAge(Animal* animal) {
return animal->age;
}
// Dog.h
typedef struct Dog Dog;
Dog* createDog(const char* name, int age, const char* breed);
void destroyDog(Dog* dog);
void dogSpeak(Animal* animal);
const char* getDogBreed(Dog* dog);
// Dog.c
#include "Dog.h"
#include "Animal.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Dog {
Animal base;
char breed[50];
};
void dogSpeak(Animal* animal) {
Dog* dog = (Dog*)animal;
printf("%s (a %s) says: Woof! Woof!\n", getAnimalName(animal), dog->breed);
}
Dog* createDog(const char* name, int age, const char* breed) {
Dog* dog = malloc(sizeof(Dog));
dog->base = *createAnimal(name, age, dogSpeak);
strncpy(dog->breed, breed, 49);
return dog;
}
void destroyDog(Dog* dog) {
destroyAnimal((Animal*)dog);
}
const char* getDogBreed(Dog* dog) {
return dog->breed;
}
// Cat.h
typedef struct Cat Cat;
Cat* createCat(const char* name, int age, const char* color);
void destroyCat(Cat* cat);
void catSpeak(Animal* animal);
const char* getCatColor(Cat* cat);
// Cat.c
#include "Cat.h"
#include "Animal.h"
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
struct Cat {
Animal base;
char color[50];
};
void catSpeak(Animal* animal) {
Cat* cat = (Cat*)animal;
printf("%s (a %s cat) says: Meow! Meow!\n", getAnimalName(animal), cat->color);
}
Cat* createCat(const char* name, int age, const char* color) {
Cat* cat = malloc(sizeof(Cat));
cat->base = *createAnimal(name, age, catSpeak);
strncpy(cat->color, color, 49);
return cat;
}
void destroyCat(Cat* cat) {
destroyAnimal((Animal*)cat);
}
const char* getCatColor(Cat* cat) {
return cat->color;
}
</stdio.h></string.h></stdlib.h></stdio.h></string.h></stdlib.h></stdio.h></string.h></stdlib.h>
이제 이걸 어떻게 사용하는지 볼까?
// main.c
#include "Animal.h"
#include "Dog.h"
#include "Cat.h"
#include <stdio.h>
void makeAnimalSpeak(Animal* animal) {
printf("The animal is about to speak: ");
animalSpeak(animal);
}
int main() {
Dog* myDog = createDog("Buddy", 3, "Labrador");
Cat* myCat = createCat("Whiskers", 2, "Tabby");
Animal* animals[] = {(Animal*)myDog, (Animal*)myCat};
int numAnimals = sizeof(animals) / sizeof(animals[0]);
for (int i = 0; i < numAnimals; i++) {
makeAnimalSpeak(animals[i]);
}
destroyDog(myDog);
destroyCat(myCat);
return 0;
}
</stdio.h>
실행 결과는 이렇게 나올 거야:
The animal is about to speak: Buddy (a Labrador) says: Woof! Woof!
The animal is about to speak: Whiskers (a Tabby cat) says: Meow! Meow!
와우! 🎉 이제 우리는 C에서도 다형성을 구현했어! makeAnimalSpeak 함수는 Animal 포인터를 받아서 animalSpeak를 호출하지만, 실제로 어떤 동물인지에 따라 다른 소리를 내는 거야. 이게 바로 다형성의 핵심이지!
🧠 Deep Dive: 이 방식은 C++의 가상 함수 테이블(vtable)과 비슷해. 각 객체가 자신의 '메서드'에 대한 포인터를 가지고 있는 거지. 실제로 많은 C++ 컴파일러가 이와 비슷한 방식으로 다형성을 구현해!
이렇게 C에서도 다형성을 구현할 수 있다니, 정말 대단하지 않아? 마치 재능넷에서 숨겨진 재능을 발견한 것 같은 기분이야! 🎭 이제 우리는 C에서도 유연하고 확장 가능한 코드를 작성할 수 있게 됐어!
6. 추상화: 복잡함을 단순하게! 🧩
추상화는 복잡한 시스템을 더 단순한 인터페이스로 표현하는 것을 말해. 객체 지향 프로그래밍에서는 주로 추상 클래스나 인터페이스를 통해 이를 구현하지. C에서는 이런 개념이 없지만, 우리가 지금까지 배운 걸 응용하면 비슷한 효과를 낼 수 있어!
예를 들어, 다양한 도형(Shape)을 다루는 프로그램을 만든다고 생각해보자. 각 도형은 면적을 계산할 수 있어야 해. 이걸 C로 어떻게 구현할 수 있을까?
// Shape.h
typedef struct Shape Shape;
Shape* createShape(double (*getArea)(Shape*), void (*destroy)(Shape*));
double shapeGetArea(Shape* shape);
void destroyShape(Shape* shape);
// Shape.c
#include "Shape.h"
#include <stdlib.h>
struct Shape {
double (*getArea)(Shape*);
void (*destroy)(Shape*);
};
Shape* createShape(double (*getArea)(Shape*), void (*destroy)(Shape*)) {
Shape* shape = malloc(sizeof(Shape));
shape->getArea = getArea;
shape->destroy = destroy;
return shape;
}
double shapeGetArea(Shape* shape) {
return shape->getArea(shape);
}
void destroyShape(Shape* shape) {
shape->destroy(shape);
}
// Circle.h
typedef struct Circle Circle;
Circle* createCircle(double radius);
void destroyCircle(Shape* shape);
double circleGetArea(Shape* shape);
// Circle.c
#include "Circle.h"
#include <stdlib.h>
#include <math.h>
#define PI 3.14159265358979323846
struct Circle {
Shape base;
double radius;
};
double circleGetArea(Shape* shape) {
Circle* circle = (Circle*)shape;
return PI * circle->radius * circle->radius;
}
void destroyCircle(Shape* shape) {
free(shape);
}
Circle* createCircle(double radius) {
Circle* circle = malloc(sizeof(Circle));
circle->base = *createShape(circleGetArea, destroyCircle);
circle->radius = radius;
return circle;
}
// Rectangle.h
typedef struct Rectangle Rectangle;
Rectangle* createRectangle(double width, double height);
void destroyRectangle(Shape* shape);
double rectangleGetArea(Shape* shape);
// Rectangle.c
#include "Rectangle.h"
#include <stdlib.h>
struct Rectangle {
Shape base;
double width;
double height;
};
double rectangleGetArea(Shape* shape) {
Rectangle* rectangle = (Rectangle*)shape;
return rectangle->width * rectangle->height;
}
void destroyRectangle(Shape* shape) {
free(shape);
}
Rectangle* createRectangle(double width, double height) {
Rectangle* rectangle = malloc(sizeof(Rectangle));
rectangle->base = *createShape(rectangleGetArea, destroyRectangle);
rectangle->width = width;
rectangle->height = height;
return rectangle;
}
</stdlib.h></math.h></stdlib.h></stdlib.h>
이제 이걸 어떻게 사용하는지 볼까?
// main.c
#include "Shape.h"
#include "Circle.h"
#include "Rectangle.h #include <stdio.h>
void printArea(Shape* shape) {
printf("The area of this shape is: %.2f\n", shapeGetArea(shape));
}
int main() {
Circle* circle = createCircle(5.0);
Rectangle* rectangle = createRectangle(4.0, 6.0);
Shape* shapes[] = {(Shape*)circle, (Shape*)rectangle};
int numShapes = sizeof(shapes) / sizeof(shapes[0]);
for (int i = 0; i < numShapes; i++) {
printArea(shapes[i]);
}
for (int i = 0; i < numShapes; i++) {
destroyShape(shapes[i]);
}
return 0;
}
</stdio.h>
실행 결과는 이렇게 나올 거야:
The area of this shape is: 78.54
The area of this shape is: 24.00
와우! 🎉 우리는 방금 C에서 추상화를 구현했어! Shape는 추상 '클래스'처럼 동작하고, Circle과 Rectangle은 이를 '상속'받아 구체적인 구현을 제공하고 있어. printArea 함수는 Shape 포인터만 알면 되고, 실제로 어떤 도형인지는 신경 쓰지 않아도 돼. 이게 바로 추상화의 힘이야!
🧠 Deep Dive: 이 패턴은 소프트웨어 설계에서 자주 사용되는 '전략 패턴(Strategy Pattern)'과 유사해. 각 도형이 자신만의 면적 계산 '전략'을 가지고 있는 거지!
이렇게 C에서도 추상화를 구현할 수 있다니, 정말 놀랍지 않아? 마치 재능넷에서 숨겨진 재능을 발견한 것 같은 기분이야! 🎭 이제 우리는 C에서도 복잡한 시스템을 단순하고 유연하게 설계할 수 있게 됐어!
7. 마무리: C의 숨겨진 매력 🌟
자, 이제 우리의 여정이 끝나가고 있어. 우리는 C 언어에서 객체 지향 프로그래밍의 주요 특징들을 어떻게 구현할 수 있는지 살펴봤어. 캡슐화, 상속, 다형성, 추상화... 이 모든 것들을 C에서 구현할 수 있다니, 정말 놀랍지 않아?
물론, 이런 방식들이 C++이나 Java처럼 언어 차원에서 지원되는 것보다는 복잡하고 번거로울 수 있어. 하지만 이를 통해 우리는 몇 가지 중요한 것들을 배웠지:
- 프로그래밍 언어의 한계는 종종 우리의 창의성으로 극복될 수 있다.
- 객체 지향 프로그래밍의 핵심 개념들은 언어에 독립적이다.
- C의 로우 레벨 특성을 이용하면 매우 유연하고 강력한 프로그래밍이 가능하다.
- 이런 기법들을 이해하면 다른 언어의 내부 동작을 더 잘 이해할 수 있다.
이 여정을 통해 우리는 C의 숨겨진 매력을 발견했어. 마치 재능넷에서 새로운 재능을 발견하는 것처럼 말이야! 🎉
🚀 Final Thought: 프로그래밍은 단순히 코드를 작성하는 것이 아니라, 문제를 해결하는 방법을 찾는 과정이야. 언어의 한계에 부딪혔을 때, 그것을 극복하는 방법을 찾는 것. 그게 바로 진정한 프로그래머의 자세지!
이제 너희들은 C를 새로운 시각으로 볼 수 있게 됐어. 앞으로 프로그래밍을 할 때, 언어의 한계에 갇히지 말고 창의적으로 문제를 해결해 나가길 바라! 그리고 기억해, 모든 언어에는 숨겨진 매력이 있다는 걸. 그 매력을 발견하는 것, 그게 바로 프로그래밍의 즐거움이야! 😊
자, 이제 너희들의 차례야. C로 멋진 객체 지향 프로그램을 만들어볼 준비가 됐니? 도전해봐! 🚀