Ionic 폼 유효성 검사 구현하기: 완벽한 가이드 📱✅
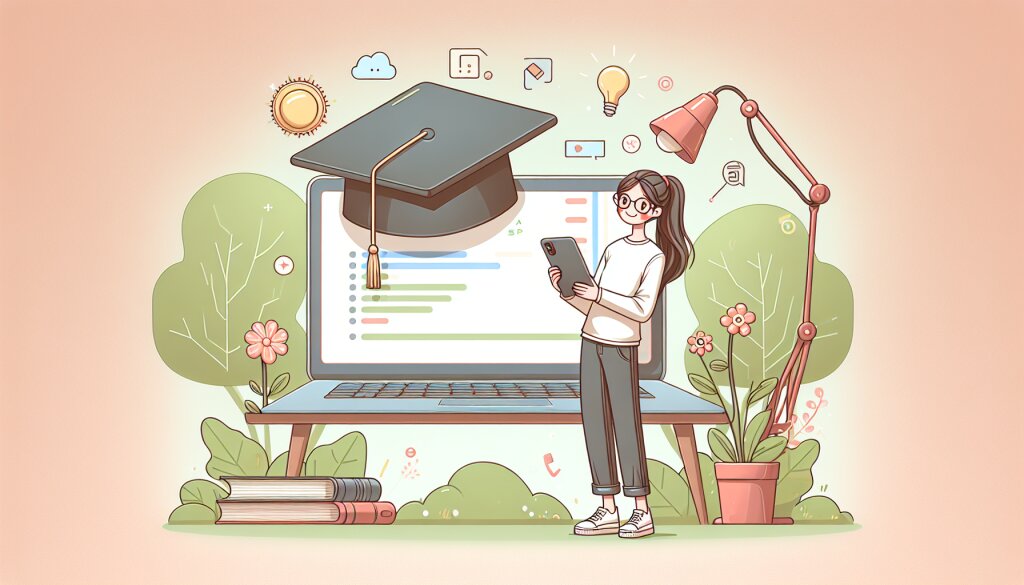
모바일 앱 개발 분야에서 폼 유효성 검사는 매우 중요한 부분입니다. 특히 Ionic 프레임워크를 사용하여 하이브리드 앱을 개발할 때, 효과적인 폼 유효성 검사 구현은 사용자 경험을 크게 향상시킬 수 있습니다. 이 글에서는 Ionic에서 폼 유효성 검사를 구현하는 방법에 대해 상세히 알아보겠습니다.
재능넷과 같은 플랫폼에서 모바일 앱 개발 관련 지식을 공유하는 것은 매우 가치 있는 일입니다. 이 글을 통해 많은 개발자들이 Ionic 폼 유효성 검사에 대한 이해를 높이고, 더 나은 앱을 만들 수 있기를 바랍니다.
1. Ionic 폼 기초 이해하기 🏗️
Ionic 프레임워크에서 폼을 다루기 위해서는 먼저 기본적인 구조와 개념을 이해해야 합니다. Ionic은 Angular를 기반으로 하고 있어, Angular의 폼 모듈을 활용합니다.
1.1 템플릿 기반 폼 vs 리액티브 폼
Ionic에서는 두 가지 주요 폼 접근 방식을 사용할 수 있습니다:
- 템플릿 기반 폼: HTML 템플릿에서 직접 폼 컨트롤을 정의하고 관리합니다.
- 리액티브 폼: 컴포넌트 클래스에서 프로그래밍 방식으로 폼 모델을 정의하고 관리합니다.
각 방식에는 장단점이 있지만, 복잡한 폼 유효성 검사를 구현할 때는 주로 리액티브 폼을 사용합니다.
1.2 Ionic 폼 컴포넌트
Ionic은 다양한 폼 관련 컴포넌트를 제공합니다:
<ion-input>
: 텍스트 입력 필드<ion-textarea>
: 여러 줄 텍스트 입력<ion-select>
: 드롭다운 선택<ion-checkbox>
: 체크박스<ion-radio>
: 라디오 버튼<ion-toggle>
: 토글 스위치
이러한 컴포넌트들은 네이티브 모바일 UI와 유사한 look and feel을 제공하여 사용자 경험을 향상시킵니다.
2. 리액티브 폼 설정하기 🛠️
리액티브 폼을 사용하여 Ionic 앱에서 폼 유효성 검사를 구현하는 과정을 살펴보겠습니다.
2.1 필요한 모듈 임포트
먼저, app.module.ts
파일에 필요한 모듈을 임포트해야 합니다:
import { ReactiveFormsModule } from '@angular/forms';
@NgModule({
imports: [
// 다른 임포트들...
ReactiveFormsModule
],
// 다른 설정들...
})
export class AppModule { }
2.2 폼 모델 정의
컴포넌트 클래스에서 FormGroup
과 FormControl
을 사용하여 폼 모델을 정의합니다:
import { FormBuilder, FormGroup, Validators } from '@angular/forms';
export class MyFormComponent implements OnInit {
myForm: FormGroup;
constructor(private formBuilder: FormBuilder) {}
ngOnInit() {
this.myForm = this.formBuilder.group({
name: ['', [Validators.required, Validators.minLength(2)]],
email: ['', [Validators.required, Validators.email]],
age: ['', [Validators.required, Validators.min(18)]],
});
}
}
여기서 FormBuilder
를 사용하여 폼 그룹을 생성하고, 각 필드에 대한 초기값과 유효성 검사 규칙을 정의했습니다.
2.3 템플릿에 폼 바인딩
HTML 템플릿에서 정의한 폼 모델을 바인딩합니다:
<form [formGroup]="myForm" (ngSubmit)="onSubmit()">
<ion-item>
<ion-label position="floating">이름</ion-label>
<ion-input formControlName="name"></ion-input>
</ion-item>
<ion-item>
<ion-label position="floating">이메일</ion-label>
<ion-input type="email" formControlName="email"></ion-input>
</ion-item>
<ion-item>
<ion-label position="floating">나이</ion-label>
<ion-input type="number" formControlName="age"></ion-input>
</ion-item>
<ion-button expand="block" type="submit" [disabled]="!myForm.valid">제출</ion-button>
</form>
formGroup
디렉티브를 사용하여 폼을 바인딩하고, 각 입력 필드에 formControlName
을 지정했습니다.
3. 유효성 검사 규칙 구현하기 🔍
Ionic 앱에서 효과적인 폼 유효성 검사를 위해 다양한 유효성 검사 규칙을 구현할 수 있습니다. Angular의 내장 Validators와 사용자 정의 Validators를 조합하여 강력한 유효성 검사 시스템을 만들 수 있습니다.
3.1 기본 유효성 검사 규칙
Angular는 다음과 같은 기본 유효성 검사 규칙을 제공합니다:
Validators.required
: 필수 입력 필드Validators.minLength(n)
: 최소 길이Validators.maxLength(n)
: 최대 길이Validators.pattern(regex)
: 정규 표현식 패턴 매칭Validators.email
: 이메일 형식 검증Validators.min(n)
: 최소값 (숫자)Validators.max(n)
: 최대값 (숫자)
이러한 기본 유효성 검사 규칙을 조합하여 사용할 수 있습니다:
this.myForm = this.formBuilder.group({
username: ['', [Validators.required, Validators.minLength(4), Validators.maxLength(20)]],
email: ['', [Validators.required, Validators.email]],
age: ['', [Validators.required, Validators.min(18), Validators.max(100)]],
password: ['', [Validators.required, Validators.minLength(8), Validators.pattern(/^(?=.*[a-z])(?=.*[A-Z])(?=.*\d)(?=.*[@$!%*?&])[A-Za-z\d@$!%*?&]{8,}$/)]],
});
3.2 사용자 정의 유효성 검사 규칙
기본 유효성 검사 규칙으로 충분하지 않은 경우, 사용자 정의 유효성 검사 규칙을 만들 수 있습니다. 예를 들어, 비밀번호 확인 필드를 검증하는 사용자 정의 유효성 검사 규칙을 만들어 보겠습니다:
import { AbstractControl, ValidationErrors, ValidatorFn } from '@angular/forms';
export function passwordMatchValidator(): ValidatorFn {
return (control: AbstractControl): ValidationErrors | null => {
const password = control.get('password');
const confirmPassword = control.get('confirmPassword');
if (password && confirmPassword && password.value !== confirmPassword.value) {
return { passwordMismatch: true };
}
return null;
};
}
// 폼 그룹에 적용
this.myForm = this.formBuilder.group({
password: ['', [Validators.required, Validators.minLength(8)]],
confirmPassword: ['', Validators.required]
}, { validators: passwordMatchValidator() });
3.3 비동기 유효성 검사
서버와의 통신이 필요한 유효성 검사의 경우, 비동기 유효성 검사를 사용할 수 있습니다. 예를 들어, 사용자명의 중복 여부를 서버에서 확인하는 경우:
import { Injectable } from '@angular/core';
import { AbstractControl, AsyncValidatorFn } from '@angular/forms';
import { Observable, of } from 'rxjs';
import { map, debounceTime, switchMap, first } from 'rxjs/operators';
@Injectable({
providedIn: 'root'
})
export class UsernameValidator {
constructor(private userService: UserService) {}
checkUsername(): AsyncValidatorFn {
return (control: AbstractControl): Observable<validationerrors null> => {
return of(control.value).pipe(
debounceTime(300),
switchMap(username => this.userService.checkUsernameAvailability(username)),
map(isAvailable => (isAvailable ? null : { usernameTaken: true })),
first()
);
};
}
}
// 폼 컨트롤에 적용
this.myForm = this.formBuilder.group({
username: ['', [Validators.required], [this.usernameValidator.checkUsername()]]
});
</validationerrors>
4. 오류 메시지 표시하기 ⚠️
사용자에게 유효성 검사 오류를 명확하게 전달하는 것은 좋은 UX를 위해 매우 중요합니다. Ionic에서는 다양한 방법으로 오류 메시지를 표시할 수 있습니다.
4.1 기본 오류 메시지 표시
가장 간단한 방법은 각 폼 필드 아래에 조건부로 오류 메시지를 표시하는 것입니다:
<ion-item>
<ion-label position="floating">이름</ion-label>
<ion-input formControlName="name"></ion-input>
</ion-item>
<ion-text color="danger" *ngIf="myForm.get('name').touched && myForm.get('name').invalid">
<p *ngIf="myForm.get('name').errors?.required">이름은 필수 입력 항목입니다.</p>
<p *ngIf="myForm.get('name').errors?.minlength">이름은 최소 2자 이상이어야 합니다.</p>
</ion-text>
4.2 동적 오류 메시지 생성
오류 메시지를 동적으로 생성하는 함수를 만들어 코드를 더 깔끔하게 관리할 수 있습니다:
getErrorMessage(fieldName: string): string {
const control = this.myForm.get(fieldName);
if (control.errors) {
if (control.errors.required) {
return `${fieldName}은(는) 필수 입력 항목입니다.`;
}
if (control.errors.minlength) {
return `${fieldName}은(는) 최소 ${control.errors.minlength.requiredLength}자 이상이어야 합니다.`;
}
if (control.errors.email) {
return '유효한 이메일 주소를 입력해주세요.';
}
// 추가 오류 유형에 대한 처리
}
return '';
}
// 템플릿에서 사용
<ion-text color="danger" *ngIf="myForm.get('name').touched && myForm.get('name').invalid">
{{ getErrorMessage('name') }}
</ion-text>
4.3 Toast 메시지 사용
Ionic의 Toast 컴포넌트를 사용하여 일시적으로 오류 메시지를 표시할 수 있습니다:
import { ToastController } from '@ionic/angular';
constructor(private toastController: ToastController) {}
async presentErrorToast(message: string) {
const toast = await this.toastController.create({
message: message,
duration: 2000,
color: 'danger',
position: 'bottom'
});
toast.present();
}
// 폼 제출 시 사용 예
onSubmit() {
if (this.myForm.valid) {
// 폼 처리 로직
} else {
this.presentErrorToast('폼에 오류가 있습니다. 다시 확인해주세요.');
}
}
4.4 Alert 사용
중요한 오류의 경우 Ionic의 Alert 컴포넌트를 사용하여 사용자의 주의를 끌 수 있습니다:
import { AlertController } from '@ionic/angular';
constructor(private alertController: AlertController) {}
async presentErrorAlert(message: string) {
const alert = await this.alertController.create({
header: '오류',
message: message,
buttons: ['확인']
});
await alert.present();
}
// 사용 예
if (this.myForm.get('password').invalid) {
this.presentErrorAlert('비밀번호는 8자 이상이어야 하며, 대문자, 소문자, 숫자, 특수문자를 포함해야 합니다.');
}
5. 폼 제출 처리하기 🚀
유효성 검사를 통과한 폼 데이터를 처리하는 것은 전체 프로세스의 마지막 단계입니다. 이 단계에서는 데이터를 서버로 전송하거나 로컬에서 처리할 수 있습니다.
5.1 폼 제출 이벤트 처리
폼 제출 이벤트를 처리하는 메서드를 구현합니다:
onSubmit() {
if (this.myForm.valid) {
console.log('폼 데이터:', this.myForm.value);
// 여기에 데이터 처리 로직 추가
this.submitFormData(this.myForm.value);
} else {
console.log('폼이 유효하지 않습니다.');
this.markFormGroupTouched(this.myForm);
}
}
// 모든 폼 컨트롤을 'touched' 상태로 만들어 오류 메시지를 표시
markFormGroupTouched(formGroup: FormGroup) {
Object.values(formGroup.controls).forEach(control => {
control.markAsTouched();
if (control instanceof FormGroup) {
this.markFormGroupTouched(control);
}
});
}
5.2 데이터 전송
유효한 폼 데이터를 서버로 전송하는 메서드를 구현합니다:
import { HttpClient } from '@angular/common/http';
import { Injectable } from '@angular/core';
@Injectable({
providedIn: 'root'
})
export class FormSubmissionService {
constructor(private http: HttpClient) {}
submitForm(data: any) {
return this.http.post('https://api.example.com/submit', data);
}
}
// 컴포넌트에서 사용
constructor(private formSubmissionService: FormSubmissionService) {}
submitFormData(data: any) {
this.formSubmissionService.submitForm(data).subscribe(
response => {
console.log('서버 응답:', response);
this.presentSuccessToast('폼이 성공적으로 제출되었습니다.');
},
error => {
console.error('오류 발생:', error);
this.presentErrorAlert('폼 제출 중 오류가 발생했습니다. 다시 시도해주세요.');
}
);
}
5.3 로딩 인디케이터 추가
데이터 전송 중 사용자에게 진행 상황을 알리기 위해 로딩 인디케이터를 추가할 수 있습니다:
import { LoadingController } from '@ionic/angular';
constructor(private loadingController: LoadingController) {}
async submitFormData(data: any) {
const loading = await this.loadingController.create({
message: '제출 중...'
});
await loading.present();
this.formSubmissionService.submitForm(data).subscribe(
response => {
loading.dismiss();
this.presentSuccessToast('폼이 성공적으로 제출되었습니다.');
},
error => {
loading.dismiss();
this.presentErrorAlert('폼 제출 중 오류가 발생했습니다. 다시 시도해주세요.');
}
);
}
5.4 폼 초기화
성공적인 제출 후 폼을 초기화하는 것이 좋습니다:
resetForm() {
this.myForm.reset();
Object.keys(this.myForm.controls).forEach(key => {
this.myForm.get(key).setErrors(null);
});
}
// 성공적인 제출 후 사용
submitFormData(data: any) {
this.formSubmissionService.submitForm(data).subscribe(
response => {
this.presentSuccessToast('폼이 성공적으로 제출되었습니다.');
this.resetForm();
},
error => {
this.presentErrorAlert('폼 제출 중 오류가 발생했습니다. 다시 시도해주세요.');
}
);
}
6. 성능 최적화 및 추가 고려사항 🔧
Ionic 앱에서 폼 유효성 검사를 구현할 때 성능 최적화와 추가적인 고려사항들이 있습니다. 이를 통해 더 나은 사용자 경험을 제공할 수 있습니다.
6.1 디바운싱(Debouncing) 구현
사용자 입력에 대한 즉각적인 유효성 검사는 성능 저하를 일으킬 수 있습니다. 디바운싱을 구현하여 이를 최적화할 수 있습니다:
import { debounceTime, distinctUntilChanged } from 'rxjs/operators';
ngOnInit() {
this.myForm.get('email').valueChanges.pipe(
debounceTime(300),
distinctUntilChanged()
).subscribe(value => {
// 여기서 유효성 검사 수행
console.log('이메일 값 변경:', value);
});
}
6.2 조건부 유효성 검사
특정 조건에 따라 유효성 검사 규칙을 동적으로 변경할 수 있습니다:
updateValidators() {
const ageControl = this.myForm.get('age');
const occupationControl = this.myForm.get('occupation');
if (ageControl.value < 18) {
occupationControl.setValidators([Validators.required]);
} else {
occupationControl.clearValidators();
}
occupationControl.updateValueAndValidity();
}
// 나이 필드의 값이 변경될 때마다 호출
this.myForm.get('age').valueChanges.subscribe(() => {
this.updateValidators();
});
6.3 국제화(i18n) 지원
다국어 지원을 위해 오류 메시지를 국제화하는 것이 좋습니다:
import { TranslateService } from '@ngx-translate/core';
constructor(private translate: TranslateService) {}
getErrorMessage(fieldName: string): string {
const control = this.myForm.get(fieldName);
if (control.errors) {
if (control.errors.required) {
return this.translate.instant('FORM_ERRORS.REQUIRED', { field: fieldName });
}
if (control.errors.minlength) {
return this.translate.instant('FORM_ERRORS.MIN_LENGTH', {
field: fieldName,
requiredLength: control.errors.minlength.requiredLength
});
}
// 추가 오류 유형에 대한 처리
}
return '';
}
6.4 접근성(Accessibility) 개선
스크린 리더 사용자를 위해 ARIA 속성을 추가하여 접근성을 개선할 수 있습니다:
<ion-item>
<ion-label position="floating">이메일</ion-label>
<ion-input
formControlName="email"
aria-describedby="email-error"
></ion-input>
</ion-item>
<ion-text color="danger" *ngIf="myForm.get('email').touched && myForm.get('email').invalid">
<p id="email-error" role="alert">{{ getErrorMessage('email') }}</p>
</ion-text>
6.5 단위 테스트 작성
폼 유효성 검사 로직에 대한 단위 테스트를 작성하여 안정성을 높일 수 있습니다:
import { TestBed, ComponentFixture } from '@angular/core/testing';
import { ReactiveFormsModule } from '@angular/forms';
import { IonicModule } from '@ionic/angular';
import { MyFormComponent } from './my-form.component';
describe('MyFormComponent', () => {
let component: MyFormComponent;
let fixture: ComponentFixture<myformcomponent>;
beforeEach(async () => {
await TestBed.configureTestingModule({
declarations: [ MyFormComponent ],
imports: [ ReactiveFormsModule, IonicModule.forRoot() ]
}).compileComponents();
fixture = TestBed.createComponent(MyFormComponent);
component = fixture.componentInstance;
fixture.detectChanges();
});
it('should create', () => {
expect(component).toBeTruthy();
});
it('should validate required fields', () => {
const nameControl = component.myForm.get('name');
nameControl.setValue('');
expect(nameControl.valid).toBeFalsy();
expect(nameControl.errors.required).toBeTruthy();
nameControl.setValue('John');
expect(nameControl.valid).toBeTruthy();
});
// 추가 테스트 케이스
});
</myformcomponent>
결론 🎉
Ionic 프레임워크를 사용한 폼 유효성 검사 구현은 사용자 경험을 크게 향상시키는 중요한 과정입니다. 이 글에서 다룬 내용을 요약하면 다음과 같습니다:
- 리액티브 폼을 사용하여 강력한 폼 구조 생성
- 다양한 유효성 검사 규칙 적용 (기본, 사용자 정의, 비동기)
- 효과적인 오류 메시지 표시 방법
- 폼 제출 처리 및 서버 통신
- 성능 최적화 기법 (디바운싱, 조건부 유효성 검사)
- 국제화 및 접근성 고려
- 단위 테스트를 통한 안정성 확보
이러한 기술과 방법을 적절히 조합하여 사용하면, 사용자 친화적이고 안정적인 Ionic 앱을 개발할 수 있습니다. 폼 유효성 검사는 단순히 데이터의 정확성을 확인하는 것을 넘어, 전체적인 앱의 품질과 사용자 만족도를 높이는 핵심 요소입니다.
앞으로도 Ionic과 Angular의 발전에 따라 더 효율적이고 혁신적인 폼 유효성 검사 방법이 등장할 것입니다. 개발자로서 이러한 변화를 주시하고, 지속적으로 학습하며 최신 기술을 적용하는 것이 중요합니다.
이 가이드가 여러분의 Ionic 앱 개발 여정에 도움이 되기를 바랍니다. 항상 사용자의 관점에서 생각하고, 끊임없이 개선하는 자세로 더 나은 앱을 만들어 나가시기 바랍니다. 행운을 빕니다! 🚀