기업용 C# 애플리케이션의 보안 강화 방법 🛡️
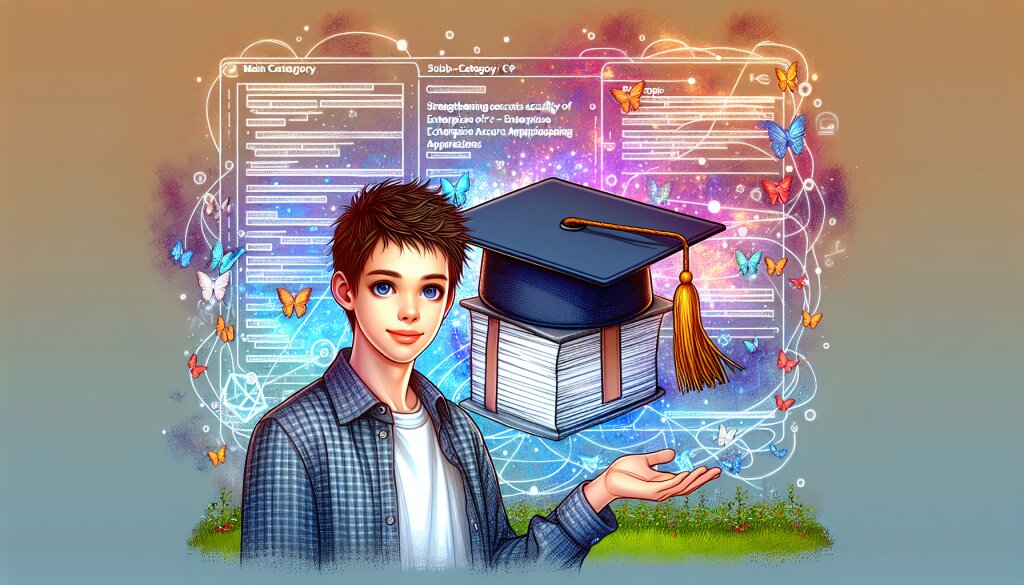
오늘날 기업 환경에서 소프트웨어 보안의 중요성은 아무리 강조해도 지나치지 않습니다. 특히 C#으로 개발된 기업용 애플리케이션의 경우, 보안 취약점으로 인한 데이터 유출이나 시스템 침해는 심각한 재정적, 평판적 손실을 초래할 수 있습니다. 이에 따라 개발자들은 보안을 최우선으로 고려하며 애플리케이션을 설계하고 구현해야 합니다.
이 글에서는 C# 기반의 기업용 애플리케이션 보안을 강화하기 위한 다양한 방법과 기술을 상세히 살펴보겠습니다. 초보 개발자부터 경험 많은 시니어 개발자까지, 모든 수준의 독자들이 실질적으로 적용할 수 있는 보안 강화 전략을 제시하고자 합니다.
재능넷과 같은 온라인 플랫폼에서 활동하는 개발자들에게도 이러한 보안 지식은 매우 중요합니다. 클라이언트의 요구사항을 충족시키면서도 안전한 애플리케이션을 제공하는 것이 전문가로서의 책임이기 때문입니다.
그럼 지금부터 C# 애플리케이션의 보안을 강화하는 방법에 대해 자세히 알아보겠습니다. 🚀
1. 입력 유효성 검사 및 데이터 검증 🔍
입력 유효성 검사는 애플리케이션 보안의 첫 번째 방어선입니다. 사용자로부터 받는 모든 입력은 잠재적인 위험을 내포하고 있으므로, 철저한 검증 과정을 거쳐야 합니다.
1.1 서버 측 유효성 검사의 중요성
클라이언트 측 유효성 검사만으로는 충분하지 않습니다. 악의적인 사용자는 클라이언트 측 검증을 우회할 수 있기 때문에, 서버 측에서 반드시 한 번 더 검증해야 합니다.
1.2 정규 표현식을 활용한 입력 검증
C#에서는 System.Text.RegularExpressions
네임스페이스의 Regex
클래스를 사용하여 강력한 입력 검증을 수행할 수 있습니다.
using System.Text.RegularExpressions;
public bool ValidateEmail(string email)
{
string pattern = @"^[\w-\.]+@([\w-]+\.)+[\w-]{2,4}$";
return Regex.IsMatch(email, pattern);
}
1.3 SQL 인젝션 방지
SQL 인젝션은 가장 위험한 보안 위협 중 하나입니다. 매개변수화된 쿼리를 사용하여 이를 방지할 수 있습니다.
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "SELECT * FROM Users WHERE Username = @Username AND Password = @Password";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@Username", username);
command.Parameters.AddWithValue("@Password", password);
// 쿼리 실행 코드...
}
1.4 크로스 사이트 스크립팅(XSS) 방지
사용자 입력을 그대로 출력하는 것은 XSS 공격의 위험을 초래합니다. 입력값을 인코딩하여 이를 방지할 수 있습니다.
using System.Web;
string userInput = "<script>alert('XSS');</script>";
string safeOutput = HttpUtility.HtmlEncode(userInput);
// 결과: <script>alert('XSS');</script>
이러한 기본적인 입력 검증 및 데이터 검증 기법들은 C# 애플리케이션의 보안을 크게 향상시킬 수 있습니다. 다음 섹션에서는 더 고급 수준의 보안 기법들을 살펴보겠습니다.
2. 암호화 및 해싱 기법 🔐
데이터 보안에 있어 암호화와 해싱은 필수적인 요소입니다. C#에서는 다양한 암호화 및 해싱 알고리즘을 제공하여 민감한 정보를 보호할 수 있습니다.
2.1 대칭 키 암호화
대칭 키 암호화는 동일한 키로 암호화와 복호화를 수행합니다. C#에서는 System.Security.Cryptography
네임스페이스의 Aes
클래스를 사용하여 구현할 수 있습니다.
using System.Security.Cryptography;
using System.Text;
public static class AesEncryption
{
public static byte[] Encrypt(string plainText, byte[] key, byte[] iv)
{
using (Aes aes = Aes.Create())
{
aes.Key = key;
aes.IV = iv;
using (MemoryStream memoryStream = new MemoryStream())
{
using (ICryptoTransform encryptor = aes.CreateEncryptor())
using (CryptoStream cryptoStream = new CryptoStream(memoryStream, encryptor, CryptoStreamMode.Write))
{
byte[] plainBytes = Encoding.UTF8.GetBytes(plainText);
cryptoStream.Write(plainBytes, 0, plainBytes.Length);
cryptoStream.FlushFinalBlock();
return memoryStream.ToArray();
}
}
}
}
public static string Decrypt(byte[] cipherText, byte[] key, byte[] iv)
{
using (Aes aes = Aes.Create())
{
aes.Key = key;
aes.IV = iv;
using (MemoryStream memoryStream = new MemoryStream(cipherText))
{
using (ICryptoTransform decryptor = aes.CreateDecryptor())
using (CryptoStream cryptoStream = new CryptoStream(memoryStream, decryptor, CryptoStreamMode.Read))
using (StreamReader streamReader = new StreamReader(cryptoStream))
{
return streamReader.ReadToEnd();
}
}
}
}
}
2.2 비대칭 키 암호화
비대칭 키 암호화는 공개 키와 개인 키를 사용하여 더 높은 수준의 보안을 제공합니다. C#에서는 RSA
클래스를 사용하여 구현할 수 있습니다.
using System.Security.Cryptography;
using System.Text;
public static class RsaEncryption
{
public static byte[] Encrypt(string plainText, RSAParameters publicKey)
{
using (RSA rsa = RSA.Create())
{
rsa.ImportParameters(publicKey);
byte[] plainBytes = Encoding.UTF8.GetBytes(plainText);
return rsa.Encrypt(plainBytes, RSAEncryptionPadding.OaepSHA256);
}
}
public static string Decrypt(byte[] cipherText, RSAParameters privateKey)
{
using (RSA rsa = RSA.Create())
{
rsa.ImportParameters(privateKey);
byte[] plainBytes = rsa.Decrypt(cipherText, RSAEncryptionPadding.OaepSHA256);
return Encoding.UTF8.GetString(plainBytes);
}
}
}
2.3 안전한 해싱 기법
패스워드와 같은 민감한 정보는 해싱을 통해 저장해야 합니다. C#에서는 SHA256
이나 HMACSHA256
과 같은 안전한 해시 알고리즘을 사용할 수 있습니다.
using System.Security.Cryptography;
using System.Text;
public static class SecureHashing
{
public static string ComputeSha256Hash(string input)
{
using (SHA256 sha256 = SHA256.Create())
{
byte[] bytes = Encoding.UTF8.GetBytes(input);
byte[] hash = sha256.ComputeHash(bytes);
return BitConverter.ToString(hash).Replace("-", "").ToLower();
}
}
}
더 안전한 패스워드 해싱을 위해서는 솔트(salt)를 추가하고 반복 횟수를 늘리는 것이 좋습니다. 이를 위해 Rfc2898DeriveBytes
클래스를 사용할 수 있습니다.
public static class PasswordHashing
{
public static string HashPassword(string password, out byte[] salt)
{
salt = new byte[16];
using (var rng = new RNGCryptoServiceProvider())
{
rng.GetBytes(salt);
}
using (var pbkdf2 = new Rfc2898DeriveBytes(password, salt, 10000))
{
byte[] hash = pbkdf2.GetBytes(20);
byte[] hashBytes = new byte[36];
Array.Copy(salt, 0, hashBytes, 0, 16);
Array.Copy(hash, 0, hashBytes, 16, 20);
return Convert.ToBase64String(hashBytes);
}
}
public static bool VerifyPassword(string password, string hashedPassword)
{
byte[] hashBytes = Convert.FromBase64String(hashedPassword);
byte[] salt = new byte[16];
Array.Copy(hashBytes, 0, salt, 0, 16);
using (var pbkdf2 = new Rfc2898DeriveBytes(password, salt, 10000))
{
byte[] hash = pbkdf2.GetBytes(20);
for (int i = 0; i < 20; i++)
{
if (hashBytes[i + 16] != hash[i])
return false;
}
return true;
}
}
}
이러한 암호화 및 해싱 기법들을 적절히 활용하면 C# 애플리케이션에서 민감한 데이터를 안전하게 보호할 수 있습니다. 다음 섹션에서는 인증 및 권한 부여에 대해 자세히 알아보겠습니다.
3. 인증 및 권한 부여 🔑
인증(Authentication)과 권한 부여(Authorization)는 애플리케이션 보안의 핵심 요소입니다. 이 두 가지 개념은 서로 다르지만 밀접하게 연관되어 있습니다.
3.1 인증 (Authentication)
인증은 사용자의 신원을 확인하는 과정입니다. C#에서는 다양한 인증 방식을 구현할 수 있습니다.
3.1.1 토큰 기반 인증
JWT(JSON Web Token)를 사용한 토큰 기반 인증은 현대적인 웹 애플리케이션에서 널리 사용됩니다. C#에서는 System.IdentityModel.Tokens.Jwt
네임스페이스를 사용하여 JWT를 생성하고 검증할 수 있습니다.
using Microsoft.IdentityModel.Tokens;
using System.IdentityModel.Tokens.Jwt;
using System.Security.Claims;
using System.Text;
public class JwtService
{
private readonly string _secret;
private readonly string _expDate;
public JwtService(IConfiguration config)
{
_secret = config.GetSection("JwtConfig").GetSection("secret").Value;
_expDate = config.GetSection("JwtConfig").GetSection("expirationInMinutes").Value;
}
public string GenerateSecurityToken(string email)
{
var tokenHandler = new JwtSecurityTokenHandler();
var key = Encoding.ASCII.GetBytes(_secret);
var tokenDescriptor = new SecurityTokenDescriptor
{
Subject = new ClaimsIdentity(new[]
{
new Claim(ClaimTypes.Email, email)
}),
Expires = DateTime.UtcNow.AddMinutes(double.Parse(_expDate)),
SigningCredentials = new SigningCredentials(new SymmetricSecurityKey(key), SecurityAlgorithms.HmacSha256Signature)
};
var token = tokenHandler.CreateToken(tokenDescriptor);
return tokenHandler.WriteToken(token);
}
}
3.1.2 다단계 인증 (MFA)
다단계 인증은 추가적인 보안 계층을 제공합니다. 예를 들어, 패스워드 입력 후 SMS로 전송된 코드를 입력하도록 요구할 수 있습니다.
public class MfaService
{
public string GenerateTwoFactorCode()
{
return new Random().Next(100000, 999999).ToString();
}
public bool ValidateTwoFactorCode(string storedCode, string inputCode)
{
return storedCode == inputCode;
}
}
3.2 권한 부여 (Authorization)
권한 부여는 인증된 사용자가 특정 리소스에 접근할 수 있는지 결정하는 과정입니다. ASP.NET Core에서는 정책 기반 권한 부여를 사용할 수 있습니다.
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthorization(options =>
{
options.AddPolicy("AdminOnly", policy => policy.RequireRole("Admin"));
options.AddPolicy("ManagerOrAdmin", policy =>
policy.RequireRole("Manager", "Admin"));
});
}
컨트롤러나 액션 메서드에 권한 정책을 적용할 수 있습니다:
[Authorize(Policy = "AdminOnly")]
public IActionResult AdminDashboard()
{
return View();
}
3.3 보안 컨텍스트 관리
C#에서는 ClaimsPrincipal
을 사용하여 현재 사용자의 보안 컨텍스트를 관리할 수 있습니다.
public class UserService
{
private readonly IHttpContextAccessor _httpContextAccessor;
public UserService(IHttpContextAccessor httpContextAccessor)
{
_httpContextAccessor = httpContextAccessor;
}
public string GetCurrentUserEmail()
{
return _httpContextAccessor.HttpContext.User.FindFirst(ClaimTypes.Email)?.Value;
}
public bool IsUserInRole(string role)
{
return _httpContextAccessor.HttpContext.User.IsInRole(role);
}
}
적절한 인증 및 권한 부여 메커니즘을 구현함으로써, C# 애플리케이션의 보안을 크게 향상시킬 수 있습니다. 다음 섹션에서는 안전한 통신 방법에 대해 알아보겠습니다.
4. 안전한 통신 프로토콜 사용 🌐
기업용 C# 애플리케이션에서 데이터 전송 시 안전한 통신 프로토콜을 사용하는 것은 매우 중요합니다. 이는 데이터 유출과 중간자 공격을 방지하는 데 필수적입니다.
4.1 HTTPS 사용
HTTPS(HTTP Secure)는 웹 통신을 암호화하는 표준 프로토콜입니다. ASP.NET Core에서는 HTTPS를 쉽게 구성할 수 있습니다.
public class Startup
{
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseHttpsRedirection();
// 다른 미들웨어 설정...
}
}
또한, 개발 환경에서 자체 서명된 인증서를 사용할 수 있습니다:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<startup>();
webBuilder.UseKestrel(options =>
{
options.Listen(IPAddress.Loopback, 5001, listenOptions =>
{
listenOptions.UseHttps("certificate.pfx", "password");
});
});
});
}
</startup>
4.2 TLS 1.2 이상 사용
TLS(Transport Layer Security)는 네트워크 통신을 암호화하는 프로토콜입니다. C#에서는 System.Net.Security
네임스페이스를 사용하여 TLS 설정을 관리할 수 있습니다.
using System.Net;
public class SecureHttpClient
{
public SecureHttpClient()
{
ServicePointManager.SecurityProtocol = SecurityProtocolType.Tls12 | SecurityProtocolType.Tls13;
}
// 클라이언트 메서드 구현...
}
4.3 안전한 웹소켓 통신
실시간 통신이 필요한 경우, 웹소켓을 사용할 수 있습니다. C#에서는 System.Net.WebSockets
네임스페이스를 사용하여 안전한 웹소켓 통신을 구현할 수 있습니다.
using System.Net.WebSockets;
public class SecureWebSocketHandler
{
private readonly ClientWebSocket _webSocket = new ClientWebSocket();
public async Task ConnectAsync(Uri uri)
{
await _webSocket.ConnectAsync(uri, CancellationToken.None);
}
public async Task SendMessageAsync(string message)
{
byte[] buffer = Encoding.UTF8.GetBytes(message);
await _webSocket.SendAsync(new ArraySegment<byte>(buffer), WebSocketMessageType.Text, true, CancellationToken.None);
}
public async Task<string> ReceiveMessageAsync()
{
var buffer = new byte[1024 * 4];
var result = await _webSocket.ReceiveAsync(new ArraySegment<byte>(buffer), CancellationToken.None);
return Encoding.UTF8.GetString(buffer, 0, result.Count);
}
}
</byte></string></byte>
4.4 API 보안
RESTful API를 사용하는 경우, API 키나 OAuth 2.0과 같은 인증 메커니즘을 구현해야 합니다.
public class ApiKeyAuthHandler : AuthenticationHandler<authenticationschemeoptions>
{
public ApiKeyAuthHandler(
IOptionsMonitor<authenticationschemeoptions> options,
ILoggerFactory logger,
UrlEncoder encoder,
ISystemClock clock)
: base(options, logger, encoder, clock)
{
}
protected override async Task<authenticateresult> HandleAuthenticateAsync()
{
if (!Request.Headers.TryGetValue("X-API-Key", out var apiKeyHeaderValues))
{
return AuthenticateResult.Fail("API Key was not provided.");
}
var providedApiKey = apiKeyHeaderValues.FirstOrDefault();
if (apiKeyIsValid(providedApiKey))
{
var claims = new[] { new Claim(ClaimTypes.Name, "API User") };
var identity = new ClaimsIdentity(claims, Scheme.Name);
var principal = new ClaimsPrincipal(identity);
var ticket = new AuthenticationTicket(principal, Scheme.Name);
return AuthenticateResult.Success(ticket);
}
return AuthenticateResult.Fail("Invalid API Key.");
}
private bool apiKeyIsValid(string providedApiKey)
{
// 실제 구현에서는 데이터베이스나 설정 파일에서 API 키를 확인해야 합니다.
var validApiKey = "your-secret-api-key";
return providedApiKey == validApiKey;
}
}
</authenticateresult></authenticationschemeoptions></authenticationschemeoptions>
이 핸들러를 사용하려면 Startup.cs
에서 다음과 같이 구성해야 합니다:
public void ConfigureServices(IServiceCollection services)
{
services.AddAuthentication("ApiKey")
.AddScheme<authenticationschemeoptions apikeyauthhandler>("ApiKey", null);
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
app.UseAuthentication();
app.UseAuthorization();
// 다른 미들웨어 설정...
}
</authenticationschemeoptions>
안전한 통신 프로토콜을 사용함으로써, 데이터 전송 중 발생할 수 있는 보안 위협을 크게 줄일 수 있습니다. 다음 섹션에서는 안전한 데이터 저장 방법에 대해 알아보겠습니다.
5. 안전한 데이터 저장 💾
기업용 C# 애플리케이션에서 데이터를 안전하게 저장하는 것은 매우 중요합니다. 이는 데이터베이스 보안, 파일 시스템 보안, 그리고 메모리 내 데이터 보호를 포함합니다.
5.1 데이터베이스 보안
데이터베이스 보안은 여러 계층에서 구현되어야 합니다:
5.1.1 연결 문자열 보호
연결 문자열은 반드시 암호화되어야 합니다. ASP.NET Core에서는 사용자 비밀(User Secrets)을 사용하여 개발 환경에서 연결 문자열을 보호할 수 있습니다:
{
"ConnectionStrings": {
"DefaultConnection": "Server=(localdb)\\mssqllocaldb;Database=MyDatabase;Trusted_Connection=True;"
}
}
프로덕션 환경에서는 환경 변수나 Azure Key Vault와 같은 안전한 저장소를 사용해야 합니다.
5.1.2 최소 권한 원칙
데이터베이스 사용자에게는 필요한 최소한의 권한만 부여해야 합니다:
-- SQL Server 예시
CREATE LOGIN AppUser WITH PASSWORD = 'ComplexPassword123!';
CREATE USER AppUser FOR LOGIN AppUser;
GRANT SELECT, INSERT, UPDATE, DELETE ON dbo.MyTable TO AppUser;
5.1.3 저장 프로시저 사용
직접적인 SQL 쿼리 대신 저장 프로시저를 사용하면 SQL 인젝션 위험을 줄일 수 있습니다:
public async Task<user> GetUserAsync(int id)
{
using (var connection = new SqlConnection(_connectionString))
{
await connection.OpenAsync();
return await connection.QuerySingleOrDefaultAsync<user>(
"sp_GetUser",
new { Id = id },
commandType: CommandType.StoredProcedure);
}
}
</user></user>
5.2 파일 시스템 보안
파일 시스템에 저장되는 데이터도 적절히 보호해야 합니다:
5.2.1 접근 제어
파일과 디렉토리에 대한 접근 권한을 제한해야 합니다:
using System.Security.AccessControl;
public void SetFilePermissions(string filePath)
{
FileSecurity fileSecurity = File.GetAccessControl(filePath);
fileSecurity.AddAccessRule(new FileSystemAccessRule("Users", FileSystemRights.Read, AccessControlType.Allow));
fileSecurity.AddAccessRule(new FileSystemAccessRule("Administrators", FileSystemRights.FullControl, AccessControlType.Allow));
File.SetAccessControl(filePath, fileSecurity);
}
5.2.2 파일 암호화
중요한 파일은 암호화하여 저장해야 합니다:
using System.Security.Cryptography;
public static void EncryptFile(string inputFile, string outputFile, byte[] key, byte[] iv)
{
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = key;
aesAlg.IV = iv;
using (FileStream fsInput = new FileStream(inputFile, FileMode.Open))
using (FileStream fsOutput = new FileStream(outputFile, FileMode.Create))
using (ICryptoTransform encryptor = aesAlg.CreateEncryptor())
using (CryptoStream csEncrypt = new CryptoStream(fsOutput, encryptor, CryptoStreamMode.Write))
{
fsInput.CopyTo(csEncrypt);
}
}
}
5.3 메모리 내 데이터 보호
메모리에 저장된 민감한 데이터도 보호해야 합니다:
5.3.1 SecureString 사용
SecureString
을 사용하여 메모리 내 문자열을 암호화할 수 있습니다:
using System.Security;
public SecureString GetSecureString(string input)
{
SecureString secure = new SecureString();
foreach (char c in input)
{
secure.AppendChar(c);
}
secure.MakeReadOnly();
return secure;
}
5.3.2 민감한 데이터 제거
민감한 데이터를 사용한 후에는 즉시 메모리에서 제거해야 합니다:
public void ProcessSensitiveData(byte[] sensitiveData)
{
try
{
// 민감한 데이터 처리
}
finally
{
Array.Clear(sensitiveData, 0, sensitiveData.Length);
}
}
안전한 데이터 저장 방법을 적용함으로써, C# 애플리케이션에서 처리하는 중요한 정보를 보호할 수 있습니다. 다음 섹션에서는 로깅과 모니터링에 대해 알아보겠습니다.
6. 로깅과 모니터링 📊
적절한 로깅과 모니터링은 보안 사고를 예방하고 대응하는 데 필수적입니다. C# 애플리케이션에서 효과적인 로깅과 모니터링을 구현하는 방법을 살펴보겠습니다.
6.1 로깅 구현
ASP.NET Core에서는 내장된 로깅 프레임워크를 사용할 수 있습니다:
public class HomeController : Controller
{
private readonly ILogger<homecontroller> _logger;
public HomeController(ILogger<homecontroller> logger)
{
_logger = logger;
}
public IActionResult Index()
{
_logger.LogInformation("Home page visited at {time}", DateTime.UtcNow);
return View();
}
[HttpPost]
public IActionResult Login(string username, string password)
{
if (IsValidUser(username, password))
{
_logger.LogInformation("User {username} logged in successfully", username);
return RedirectToAction("Dashboard");
}
else
{
_logger.LogWarning("Failed login attempt for user {username}", username);
return View("LoginFailed");
}
}
}
</homecontroller></homecontroller>
6.2 보안 이벤트 로깅
보안 관련 이벤트는 특별히 주의하여 로깅해야 합니다:
public class SecurityEventLogger
{
private readonly ILogger<securityeventlogger> _logger;
public SecurityEventLogger(ILogger<securityeventlogger> logger)
{
_logger = logger;
}
public void LogSecurityEvent(string eventType, string description, string userId = null)
{
var logMessage = new
{
EventType = eventType,
Description = description,
UserId = userId,
Timestamp = DateTime.UtcNow
};
_logger.LogWarning("Security Event: {@SecurityEvent}", logMessage);
}
}
</securityeventlogger></securityeventlogger>
6.3 로그 보안
로그 자체도 보안의 대상이 되어야 합니다:
public class Program
{
public static void Main(string[] args)
{
CreateHostBuilder(args).Build().Run();
}
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureLogging(logging =>
{
logging.ClearProviders();
logging.AddFile("Logs/myapp-{Date}.txt", LogLevel.Warning);
logging.AddEventLog(new EventLogSettings
{
SourceName = "MyApp",
LogName = "Application"
});
})
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<startup>();
});
}
</startup>
6.4 모니터링 구현
애플리케이션 성능 및 보안 모니터링을 위해 Application Insights와 같은 도구를 사용할 수 있습니다:
public void ConfigureServices(IServiceCollection services)
{
services.AddApplicationInsightsTelemetry();
}
그리고 컨트롤러에서 다음과 같이 사용할 수 있습니다:
public class HomeController : Controller
{
private readonly TelemetryClient _telemetryClient;
public HomeController(TelemetryClient telemetryClient)
{
_telemetryClient = telemetryClient;
}
public IActionResult Index()
{
_telemetryClient.TrackEvent("HomePageVisited");
return View();
}
}
6.5 알림 설정
중요한 보안 이벤트가 발생했을 때 즉시 알림을 받을 수 있도록 설정해야 합니다:
public class SecurityAlertService
{
private readonly ILogger<securityalertservice> _logger;
private readonly IEmailService _emailService;
public SecurityAlertService(ILogger<securityalertservice> logger, IEmailService emailService)
{
_logger = logger;
_emailService = emailService;
}
public async Task SendAlertAsync(string alertMessage)
{
_logger.LogCritical("Security Alert: {AlertMessage}", alertMessage);
await _emailService.SendEmailAsync("security@company.com", "Security Alert", alertMessage);
}
}
</securityalertservice></securityalertservice>
효과적인 로깅과 모니터링 시스템을 구축함으로써, 보안 위협을 신속하게 감지하고 대응할 수 있습니다. 이는 C# 애플리케이션의 전반적인 보안 상태를 크게 향상시킵니다.