TypeScript Compiler API 활용하기: 강력한 도구의 세계로 🚀
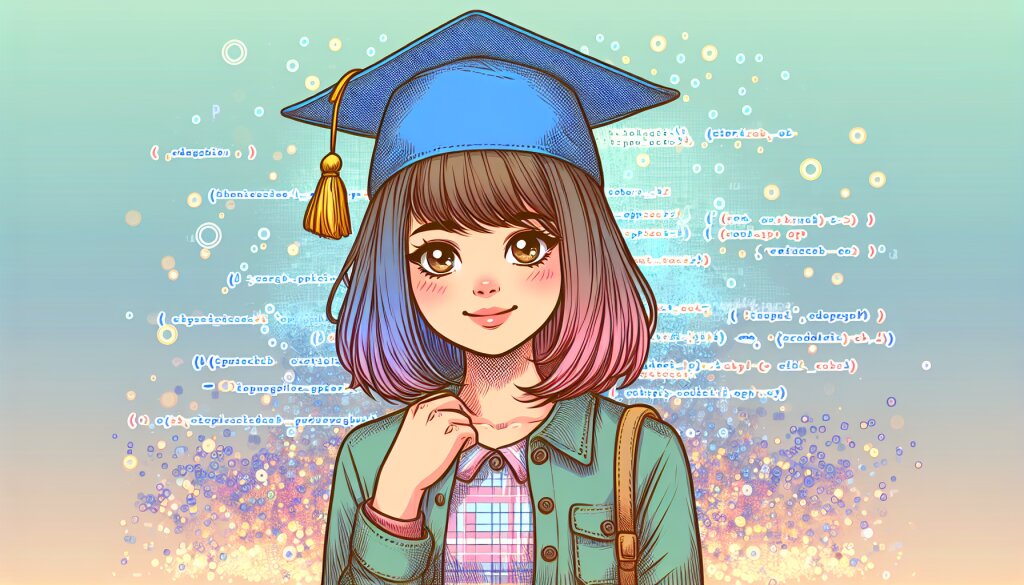
TypeScript는 현대 웹 개발에서 필수적인 도구로 자리 잡았습니다. 특히 대규모 프로젝트에서 그 진가를 발휘하죠. 그런데 TypeScript의 진정한 힘은 단순히 정적 타입 체킹에만 국한되지 않습니다. TypeScript Compiler API를 활용하면 코드 분석, 변환, 생성 등 다양한 작업을 수행할 수 있어요. 이는 마치 재능넷에서 다양한 재능을 거래하듯, 개발자들에게 새로운 가능성의 세계를 열어줍니다.
이 글에서는 TypeScript Compiler API의 심도 있는 활용법을 탐구해볼 예정입니다. 기본 개념부터 시작해 고급 기술까지, 단계별로 살펴보겠습니다. 준비되셨나요? 함께 TypeScript의 숨겨진 보물을 찾아 떠나봅시다! 🗺️
1. TypeScript Compiler API 소개 🌟
TypeScript Compiler API는 TypeScript 컴파일러의 내부 동작에 접근할 수 있게 해주는 강력한 도구입니다. 이를 통해 개발자는 TypeScript 코드를 프로그래밍 방식으로 분석하고, 변환하고, 생성할 수 있습니다.
이 API의 주요 특징은 다음과 같습니다:
- 코드 분석: 추상 구문 트리(AST)를 통해 코드 구조를 깊이 있게 분석할 수 있습니다.
- 코드 변환: 기존 코드를 새로운 형태로 변환할 수 있습니다.
- 코드 생성: 프로그래밍 방식으로 새로운 TypeScript 코드를 생성할 수 있습니다.
- 타입 체킹: 코드의 타입 정보를 프로그래밍 방식으로 확인할 수 있습니다.
이러한 기능들은 코드 리팩토링 도구, 린트 도구, 코드 생성기 등 다양한 개발 도구를 만드는 데 활용될 수 있습니다.
TypeScript Compiler API를 사용하면 코드를 더 깊이 이해하고 조작할 수 있게 됩니다. 이는 마치 숙련된 장인이 자신의 도구를 자유자재로 다루는 것과 같죠. 이제 우리도 이 강력한 도구를 어떻게 활용할 수 있는지 자세히 알아보겠습니다.
2. TypeScript Compiler API의 기본 구조 🏗️
TypeScript Compiler API를 효과적으로 사용하기 위해서는 그 기본 구조를 이해하는 것이 중요합니다. API는 여러 모듈로 구성되어 있으며, 각 모듈은 특정 기능을 담당합니다.
주요 모듈은 다음과 같습니다:
- ts.createProgram: TypeScript 프로그램을 생성합니다.
- ts.createSourceFile: 소스 파일을 생성합니다.
- ts.createPrinter: AST를 문자열로 변환합니다.
- ts.createCompilerHost: 컴파일러 호스트를 생성합니다.
- ts.getPreEmitDiagnostics: 컴파일 전 진단 정보를 얻습니다.
이러한 모듈들을 조합하여 다양한 작업을 수행할 수 있습니다. 예를 들어, 코드를 분석하고 싶다면 ts.createProgram
과 ts.createSourceFile
을 사용하여 AST를 생성한 후, 이를 순회하며 원하는 정보를 추출할 수 있죠.
이 구조를 이해하고 나면, TypeScript Compiler API를 사용하여 복잡한 작업을 수행하는 것이 훨씬 쉬워집니다. 마치 퍼즐의 각 조각을 이해하고 나면 전체 그림을 완성하기가 쉬워지는 것처럼 말이죠.
다음 섹션에서는 이러한 기본 구조를 바탕으로 실제 코드 예제를 통해 API의 사용법을 자세히 살펴보겠습니다. 🧩
3. TypeScript Compiler API 시작하기 🚀
TypeScript Compiler API를 사용하기 위한 첫 걸음을 떼어봅시다. 먼저, 필요한 모듈을 설치하고 기본적인 설정을 해야 합니다.
3.1 환경 설정
TypeScript와 필요한 타입 정의를 설치합니다:
npm install typescript @types/node
그리고 tsconfig.json
파일을 생성하여 기본 설정을 합니다:
{
"compilerOptions": {
"target": "es2018",
"module": "commonjs",
"strict": true,
"esModuleInterop": true,
"outDir": "./dist",
"rootDir": "./src"
}
}
3.2 기본 예제: 소스 파일 생성하기
이제 TypeScript Compiler API를 사용하여 간단한 소스 파일을 생성해봅시다.
import * as ts from 'typescript';
function createSourceFile() {
const sourceText = `
function greet(name: string) {
console.log(\`Hello, \${name}!\`);
}
greet('TypeScript');
`;
const sourceFile = ts.createSourceFile(
'example.ts',
sourceText,
ts.ScriptTarget.Latest,
/*setParentNodes*/ true
);
return sourceFile;
}
const sourceFile = createSourceFile();
console.log(sourceFile.getText());
이 예제에서는 ts.createSourceFile
함수를 사용하여 간단한 TypeScript 코드를 포함하는 소스 파일을 생성합니다. 이렇게 생성된 소스 파일은 추후 분석이나 변환에 사용될 수 있습니다.
이 기본 예제를 통해 TypeScript Compiler API의 기본적인 사용법을 익힐 수 있습니다. 다음 섹션에서는 더 복잡한 작업을 수행하는 방법을 알아보겠습니다. 🎓
4. AST(추상 구문 트리) 탐색하기 🌳
AST(Abstract Syntax Tree)는 소스 코드의 구조를 트리 형태로 표현한 것입니다. TypeScript Compiler API를 사용하면 이 AST를 탐색하고 조작할 수 있습니다. 이는 코드 분석, 변환, 최적화 등 다양한 작업에 활용될 수 있죠.
4.1 AST 생성하기
먼저, 소스 코드로부터 AST를 생성해봅시다:
import * as ts from 'typescript';
function createAST(sourceCode: string): ts.SourceFile {
return ts.createSourceFile(
'example.ts',
sourceCode,
ts.ScriptTarget.Latest,
true
);
}
const sourceCode = `
function greet(name: string) {
console.log(\`Hello, \${name}!\`);
}
greet('TypeScript');
`;
const ast = createAST(sourceCode);
4.2 AST 탐색하기
이제 생성된 AST를 탐색해봅시다. 예를 들어, 모든 함수 선언을 찾아보겠습니다:
function findFunctionDeclarations(node: ts.Node) {
if (ts.isFunctionDeclaration(node)) {
console.log(`Found function: ${node.name?.getText()}`);
}
ts.forEachChild(node, findFunctionDeclarations);
}
findFunctionDeclarations(ast);
이 코드는 AST를 순회하면서 모든 함수 선언을 찾아 출력합니다.
AST를 탐색하는 것은 마치 거대한 나무의 가지를 따라 올라가는 것과 같습니다. 각 노드는 코드의 특정 부분을 나타내며, 이를 통해 코드의 구조를 깊이 있게 이해할 수 있습니다.
4.3 AST 변환하기
AST를 변환하여 코드를 수정할 수도 있습니다. 예를 들어, 모든 함수 이름을 대문자로 바꿔봅시다:
function transformFunctionNames(context: ts.TransformationContext) {
return (rootNode: ts.SourceFile) => {
function visit(node: ts.Node): ts.Node {
if (ts.isFunctionDeclaration(node) && node.name) {
return ts.factory.updateFunctionDeclaration(
node,
node.decorators,
node.modifiers,
node.asteriskToken,
ts.factory.createIdentifier(node.name.text.toUpperCase()),
node.typeParameters,
node.parameters,
node.type,
node.body
);
}
return ts.visitEachChild(node, visit, context);
}
return ts.visitNode(rootNode, visit);
};
}
const result = ts.transform(ast, [transformFunctionNames]);
const printer = ts.createPrinter();
console.log(printer.printFile(result.transformed[0] as ts.SourceFile));
이 코드는 AST를 변환하여 모든 함수 이름을 대문자로 바꾸고, 그 결과를 출력합니다.
AST 탐색과 변환은 TypeScript Compiler API의 핵심 기능 중 하나입니다. 이를 통해 코드 분석 도구, 자동 리팩토링 도구, 커스텀 린트 규칙 등 다양한 개발 도구를 만들 수 있습니다. 마치 재능넷에서 다양한 재능을 발견하고 활용하듯, AST를 통해 코드의 새로운 가능성을 발견할 수 있죠. 🌟
5. 타입 체커 활용하기 🔍
TypeScript Compiler API의 강력한 기능 중 하나는 타입 체커입니다. 이를 통해 코드의 타입 정보를 프로그래밍 방식으로 확인하고 활용할 수 있습니다.
5.1 타입 체커 생성하기
먼저, 타입 체커를 생성해봅시다:
import * as ts from 'typescript';
function createTypeChecker(files: { [fileName: string]: string }) {
const compilerHost = ts.createCompilerHost({});
compilerHost.readFile = (fileName: string) => files[fileName];
compilerHost.getSourceFile = (fileName: string, languageVersion: ts.ScriptTarget) => {
const sourceText = files[fileName];
return sourceText !== undefined
? ts.createSourceFile(fileName, sourceText, languageVersion)
: undefined;
};
const program = ts.createProgram(Object.keys(files), {}, compilerHost);
return program.getTypeChecker();
}
const files = {
'example.ts': `
function greet(name: string) {
return \`Hello, \${name}!\`;
}
const result = greet(42);
`
};
const typeChecker = createTypeChecker(files);
5.2 타입 정보 확인하기
이제 생성된 타입 체커를 사용하여 코드의 타입 정보를 확인해봅시다:
function checkTypes(sourceFile: ts.SourceFile) {
function visit(node: ts.Node) {
if (ts.isCallExpression(node)) {
const signature = typeChecker.getResolvedSignature(node);
if (signature) {
console.log(`Function call at position ${node.pos}:`);
console.log(` Return type: ${typeChecker.typeToString(signature.getReturnType())}`);
signature.parameters.forEach((param, index) => {
const paramType = typeChecker.getTypeOfSymbolAtLocation(param, node.arguments[index] || node);
console.log(` Parameter ${index} type: ${typeChecker.typeToString(paramType)}`);
});
}
}
ts.forEachChild(node, visit);
}
visit(sourceFile);
}
const sourceFile = ts.createSourceFile('example.ts', files['example.ts'], ts.ScriptTarget.Latest, true);
checkTypes(sourceFile);
이 코드는 함수 호출의 반환 타입과 매개변수 타입을 확인하고 출력합니다.
5.3 타입 오류 감지하기
타입 체커를 사용하여 코드의 타입 오류를 감지할 수도 있습니다:
function getDiagnostics(program: ts.Program) {
const diagnostics = ts.getPreEmitDiagnostics(program);
return diagnostics.map(diagnostic => {
if (diagnostic.file) {
const { line, character } = diagnostic.file.getLineAndCharacterOfPosition(diagnostic.start!);
const message = ts.flattenDiagnosticMessageText(diagnostic.messageText, '\n');
return `${diagnostic.file.fileName} (${line + 1},${character + 1}): ${message}`;
} else {
return ts.flattenDiagnosticMessageText(diagnostic.messageText, '\n');
}
});
}
const program = ts.createProgram(Object.keys(files), {}, ts.createCompilerHost({}));
const diagnostics = getDiagnostics(program);
console.log(diagnostics);
이 코드는 프로그램의 모든 타입 오류를 감지하고 출력합니다.
타입 체커를 활용하면 코드의 타입 안정성을 프로그래밍 방식으로 확인하고 개선할 수 있습니다. 이는 대규모 프로젝트에서 특히 유용하며, 자동화된 코드 리뷰 도구나 IDE 플러그인 개발 등에 활용될 수 있습니다. 마치 재능넷에서 전문가의 조언을 받듯, 타입 체커는 우리 코드의 품질을 높이는 든든한 조력자 역할을 합니다. 💪
6. 코드 생성과 변환 🔄
TypeScript Compiler API를 사용하면 새로운 코드를 생성하거나 기존 코드를 변환할 수 있습니다. 이는 코드 리팩토링, 자동 문서화, 코드 최적화 등 다양한 용도로 활용될 수 있습니다.
6.1 코드 생성하기
먼저, TypeScript Compiler API를 사용하여 새로운 코드를 생성해봅시다:
import * as ts from 'typescript';
function generateHelloWorld() {
const functionDeclaration = ts.factory.createFunctionDeclaration(
undefined,
undefined,
undefined,
ts.factory.createIdentifier("helloWorld"),
undefined,
[],
undefined,
ts.factory.createBlock([
ts.factory.createExpressionStatement(
ts.factory.createCallExpression(
ts.factory.createPropertyAccessExpression(
ts.factory.createIdentifier("console"),
ts.factory.createIdentifier("log")
),
undefined,
[ts.factory.createStringLiteral("Hello, World!")]
)
)
])
);
const sourceFile = ts.createSourceFile("generated.ts", "", ts.ScriptTarget.Latest, false, ts.ScriptKind.TS);
const printer = ts.createPrinter({ newLine: ts.NewLineKind.LineFeed });
return printer.printNode(ts.EmitHint.Unspecified, functionDeclaration, sourceFile);
}
console.log(generateHelloWorld());
이 코드는 "Hello, World!"를 출력하는 함수를 프로그래밍 방식으로 생성합니다.
6.2 코드 변환하기
다음으로, 기존 코드를 변환하는 예제를 살펴보겠습니다. 이 예제에서는 모든 변수 선언을 const로 변경합니다:
import * as ts from 'typescript';
function transformVariablesToConst(context: ts.TransformationContext) {
return (rootNode: ts.SourceFile) => {
function visit(node: ts.Node): ts.Node {
if (ts.isVariableStatement(node)) {
const newDeclarationList = ts.factory.updateVariableDeclarationList(
node.declarationList,
node.declarationList.declarations,
ts.NodeFlags.Const
);
return ts.factory.updateVariableStatement(
node,
node.modifiers,
newDeclarationList
);
}
return ts.visitEachChild(node, visit, context);
}
return ts.visitNode(rootNode, visit);
};
}
const sourceCode = `
let x = 5;
var y = 10;
const z = 15;
`;
const sourceFile = ts.createSourceFile("example.ts", sourceCode, ts.ScriptTarget.Latest, true);
const result = ts.transform(sourceFile, [transformVariablesToConst]);
const printer = ts.createPrinter();
console.log(printer.printFile(result.transformed[0] as ts.SourceFile));
이 코드는 모든 let과 var 선언을 const로 변환합니다.
코드 생성과 변환은 TypeScript Compiler API의 강력한 기능 중 하나입니다. 이를 통해 코드 리팩토링 도구, 코드 최적화 도구, 커스텀 트랜스파일러 등을 만들 수 있습니다. 마치 재능넷에서 다양한 재능을 조합하여 새로운 가치를 창출하듯, 코드 생성과 변환을 통해 우리는 프로그래밍의 새로운 가능성을 열어갈 수 있습니다. 🚀
7. 실제 프로젝트에서의 활용 사례 💼
TypeScript Compiler API는 다양한 실제 프로젝트에서 활용되고 있습니다. 몇 가지 대표적인 사례를 살펴보겠습니다.
7.1 커스텀 린트 규칙 만들기
ESLint와 같은 린트 도구에 커스텀 규칙을 추가할 때 TypeScript Compiler API를 활용할 수 있습니다.
import * as ts from 'typescript';
function noVarRule(context: any) {
return {
VariableDeclaration(node: any) {
if (node.kind === ts.SyntaxKind.VariableDeclaration) {
const declaration = node as ts.VariableDeclaration;
if (declaration.parent && declaration.parent.kind === ts.SyntaxKind.VariableDeclarationList) {
const declarationList = declaration.parent as ts.VariableDeclarationList;
if (declarationList.flags & ts.NodeFlags.Let) {
context.report({
node,
message: "Use 'const' instead of 'let' when possible.",
});
}
}
}
}
};
}
이 예제는 'let' 대신 'const'를 사용하도록 권장하는 린트 규칙을 만듭니다.
7.2 자동 문서화 도구 개발
TypeScript Compiler API를 사용하여 코드의 구조를 분석하고 자동으로 문서를 생성할 수 있습니다.
import * as ts from 'typescript';
function generateDocumentation(sourceFile: ts.SourceFile) {
let documentation = '';
function visit(node: ts.Node) {
if (ts.isFunctionDeclaration(node) && node.name) {
documentation += `\n## Function: ${node.name.text}\n`;
if (node.jsDoc) {
documentation += node.jsDoc.map(doc => doc.comment).join('\n');
}
documentation += '\n\nParameters:\n';
node.parameters.forEach(param => {
documentation += `- ${param.name.getText()}: ${param.type ? param.type.getText() : 'any'}\n`;
});
}
ts.forEachChild(node, visit);
}
visit(sourceFile);
return documentation;
}
const sourceCode = `
/**
* Greets a person
* @param name The name of the person
* @returns A greeting message
*/
function greet(name: string): string {
return \`Hello, \${name}!\`;
}
`;
const sourceFile = ts.createSourceFile("example.ts", sourceCode, ts.ScriptTarget.Latest, true);
console.log(generateDocumentation(sourceFile));
이 코드는 함수 선언을 분석하여 자동으로 문서를 생성합니다.
7.3 코드 리팩토링 도구 개발
TypeScript Compiler API를 사용하여 대규모 코드베이스를 자동으로 리팩토링할 수 있습니다.
import * as ts from 'typescript';
function renameVariable(sourceFile: ts.SourceFile, oldName: string, newName: string) {
function visit(node: ts.Node): ts.Node {
if (ts.isIdentifier(node) && node.text === oldName) {
return ts.factory.createIdentifier(newName);
}
return ts.visitEachChild(node, visit, ts.nullTransformationContext);
}
return ts.visitNode(sourceFile, visit);
}
const sourceCode = `
let x = 5;
console.log(x);
`;
const sourceFile = ts.createSourceFile("example.ts", sourceCode, ts.ScriptTarget.Latest, true);
const result = renameVariable(sourceFile, 'x', 'myVariable');
const printer = ts.createPrinter();
console.log(printer.printFile(result as ts.SourceFile));
이 코드는 변수 이름을 자동으로 변경하는 리팩토링 도구의 예시입니다.
이러한 활용 사례들은 TypeScript Compiler API의 강력함을 보여줍니다. 이 API를 통해 우리는 코드를 더 깊이 이해하고, 더 효율적으로 관리할 수 있게 됩니다. 마치 재능넷에서 다양한 전문가들의 재능을 활용하여 복잡한 프로젝트를 수행하듯, TypeScript Compiler API는 우리에게 코드를 다루는 새로운 방법을 제시합니다. 🌟
8. 결론 및 향후 전망 🔮
지금까지 우리는 TypeScript Compiler API의 다양한 측면을 살펴보았습니다. 이 강력한 도구는 코드 분석, 변환, 생성 등 다양한 작업을 가능하게 하며, 개발자들에게 코드를 다루는 새로운 방법을 제시합니다.
8.1 주요 이점 정리
- 코드 이해도 향상: AST를 통해 코드 구조를 깊이 이해할 수 있습니다.
- 자동화 가능성: 반복적인 코드 작업을 자동화할 수 있습니다.
- 타입 안정성: 타입 체커를 통해 코드의 타입 안정성을 프로그래밍 방식으로 확인할 수 있습니다.
- 도구 개발: 린트, 리팩토링, 문서화 등 다양한 개발 도구를 만들 수 있습니다.
8.2 향후 전망
TypeScript Compiler API의 미래는 밝아 보입니다. 앞으로 더 많은 개발자들이 이 도구를 활용하여 혁신적인 개발 도구와 워크플로우를 만들어낼 것으로 예상됩니다. 특히 다음과 같은 영역에서 발전이 기대됩니다:
- AI 기반 코드 분석: TypeScript Compiler API와 AI를 결합하여 더 지능적인 코드 분석 도구를 만들 수 있을 것입니다.
- 실시간 코드 최적화: 개발 중인 코드를 실시간으로 분석하고 최적화하는 도구가 등장할 수 있습니다.
- 크로스 플랫폼 도구: 다양한 프로그래밍 언어와 플랫폼을 아우르는 통합 개발 도구가 만들어질 수 있습니다.
TypeScript Compiler API는 개발자들에게 코드를 다루는 새로운 방법을 제시합니다. 이는 마치 재능넷에서 다양한 재능을 발견하고 조합하여 새로운 가치를 창출하는 것과 같습니다. 앞으로 이 강력한 도구를 활용하여 더 나은 개발 경험을 만들어나갈 수 있기를 기대합니다.
TypeScript Compiler API의 세계는 아직 많은 가능성을 품고 있습니다. 우리는 이제 막 그 가능성의 문턱에 서 있을 뿐입니다. 앞으로 더 많은 개발자들이 이 도구를 탐험하고, 새로운 아이디어를 실현시켜 나갈 것입니다. 그 과정에서 우리는 프로그래밍의 새로운 지평을 열어갈 수 있을 것입니다. 함께 이 흥미진진한 여정을 계속해 나가봅시다! 🚀