Unity 모바일 게임 세이브 시스템 구현 🎮💾
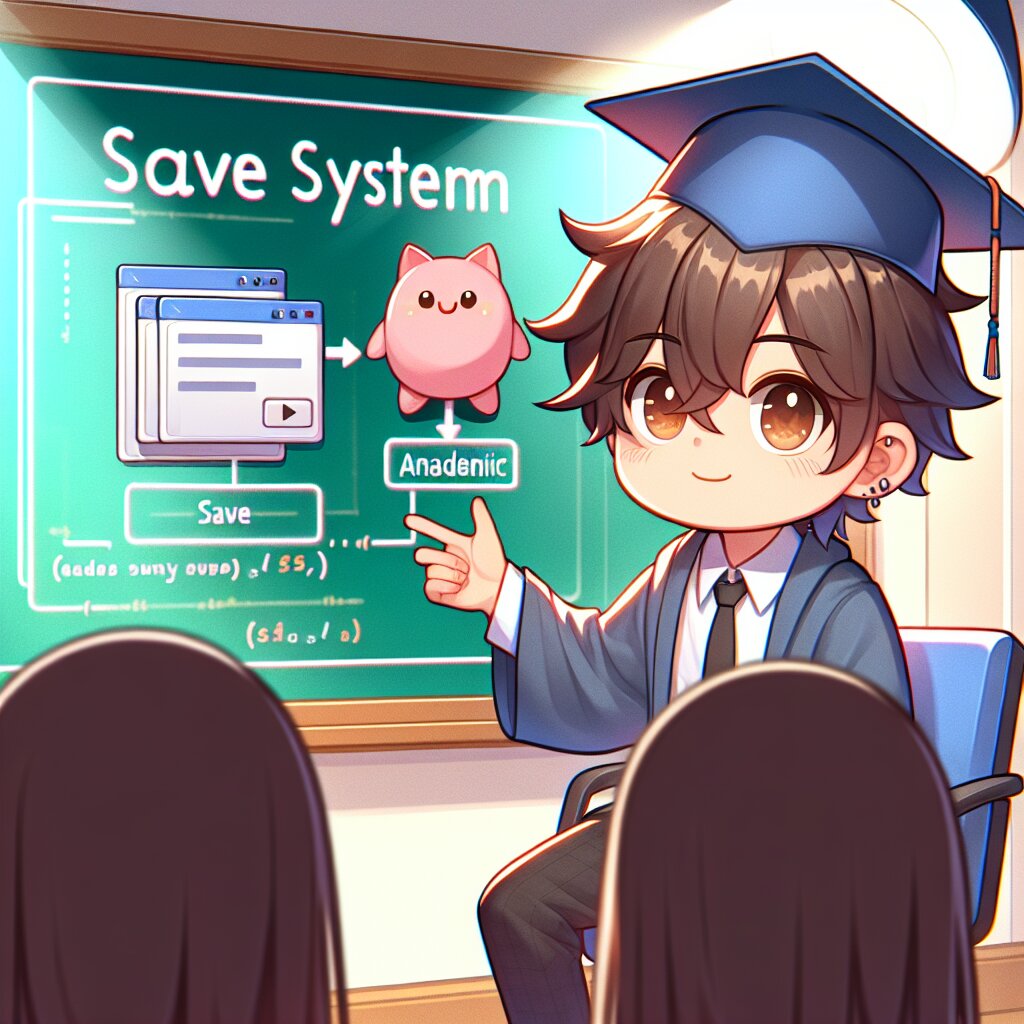
안녕, 게임 개발자 친구들! 오늘은 Unity로 모바일 게임을 만들 때 정말 중요한 주제인 '세이브 시스템 구현'에 대해 재미있게 얘기해볼 거야. 🚀 이 글을 읽고 나면 너도 게임 데이터를 저장하고 불러오는 마법사가 될 수 있을 거야! 😉
잠깐! 이 글은 재능넷(https://www.jaenung.net)의 '지식인의 숲' 메뉴에서 볼 수 있어. 재능넷은 다양한 재능을 거래하는 플랫폼이니, 게임 개발 관련 도움이 필요하다면 한 번 들러봐! 👀
자, 이제 본격적으로 시작해볼까? 🏁
1. 세이브 시스템이 왜 중요할까? 🤔
게임을 만들 때 가장 중요한 건 뭘까? 그래픽? 사운드? 아니면 재미있는 게임플레이? 다 맞는 말이지만, 플레이어의 진행 상황을 저장하는 것도 엄청 중요해! 왜 그런지 한번 생각해볼까?
- 📱 모바일 게임은 언제 어디서나 할 수 있어야 해
- 🔋 배터리가 떨어지거나 갑자기 앱이 종료되더라도 진행 상황이 날아가면 안 돼
- 🏆 플레이어의 업적이나 레벨 같은 중요한 정보를 기억해야 해
- 💰 인앱 구매 정보도 안전하게 저장해야 해
이런 이유들 때문에 세이브 시스템은 게임에서 절대 빠질 수 없는 핵심 기능이야. 재능넷에서도 게임 개발 관련 재능을 거래할 때 세이브 시스템 구현 능력은 정말 중요하게 여겨진다고 해. 그만큼 중요하다는 거지! 😎
주의사항: 세이브 시스템을 제대로 구현하지 않으면 플레이어들이 게임을 금방 지워버릴 수 있어. 그러니까 정말 신경 써서 만들어야 해!
자, 이제 Unity에서 어떻게 세이브 시스템을 구현하는지 하나씩 알아볼까? 🕵️♂️
2. Unity에서 사용할 수 있는 데이터 저장 방식 🗃️
Unity에서는 여러 가지 방법으로 데이터를 저장할 수 있어. 각각의 방식에는 장단점이 있으니, 상황에 맞게 선택해서 사용하면 돼. 어떤 방식들이 있는지 한번 살펴볼까?
2.1 PlayerPrefs 🍪
PlayerPrefs는 Unity에서 제공하는 가장 간단한 데이터 저장 방식이야. 마치 웹브라우저의 쿠키같은 거라고 생각하면 돼.
- 👍 장점: 사용하기 쉽고, 간단한 데이터를 빠르게 저장할 수 있어.
- 👎 단점: 보안이 취약하고, 복잡한 데이터 구조를 저장하기 어려워.
PlayerPrefs를 사용하는 간단한 예제를 볼까?
// 데이터 저장하기
PlayerPrefs.SetInt("Score", 100);
PlayerPrefs.SetString("PlayerName", "Unity마스터");
PlayerPrefs.Save();
// 데이터 불러오기
int score = PlayerPrefs.GetInt("Score", 0); // 기본값 0
string playerName = PlayerPrefs.GetString("PlayerName", "NoName"); // 기본값 "NoName"
PlayerPrefs는 간단한 설정이나 작은 양의 데이터를 저장할 때 유용해. 하지만 중요한 게임 데이터나 보안이 필요한 정보는 다른 방식으로 저장하는 게 좋아.
2.2 JSON 직렬화 📄
JSON(JavaScript Object Notation)은 데이터를 저장하고 전송하는 데 많이 사용되는 형식이야. Unity에서도 JSON을 이용해 데이터를 저장할 수 있어.
- 👍 장점: 복잡한 데이터 구조도 쉽게 저장할 수 있고, 가독성이 좋아.
- 👎 단점: PlayerPrefs보다는 조금 더 복잡하고, 암호화가 필요할 수 있어.
JSON을 사용한 데이터 저장 예제를 볼까?
using UnityEngine;
using System.IO;
[System.Serializable]
public class PlayerData
{
public string playerName;
public int level;
public float health;
}
public class SaveSystem : MonoBehaviour
{
public void SavePlayerData(PlayerData data)
{
string json = JsonUtility.ToJson(data);
File.WriteAllText(Application.persistentDataPath + "/playerData.json", json);
}
public PlayerData LoadPlayerData()
{
string path = Application.persistentDataPath + "/playerData.json";
if (File.Exists(path))
{
string json = File.ReadAllText(path);
return JsonUtility.FromJson<playerdata>(json);
}
else
{
Debug.LogError("Save file not found in " + path);
return null;
}
}
}
</playerdata>
JSON은 구조화된 데이터를 저장하기에 좋은 방식이야. 게임의 세이브 데이터처럼 여러 정보를 한꺼번에 저장해야 할 때 유용해.
2.3 바이너리 직렬화 💾
바이너리 직렬화는 데이터를 이진 형식으로 변환해서 저장하는 방식이야. 텍스트 기반의 JSON보다 더 효율적이고 빠르게 데이터를 처리할 수 있어.
- 👍 장점: 저장 공간을 적게 차지하고, 처리 속도가 빨라.
- 👎 단점: 사람이 직접 읽기 어렵고, 플랫폼 간 호환성 문제가 있을 수 있어.
바이너리 직렬화를 사용한 예제를 살펴볼까?
using UnityEngine;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[System.Serializable]
public class PlayerData
{
public string playerName;
public int level;
public float health;
}
public class SaveSystem : MonoBehaviour
{
public void SavePlayerData(PlayerData data)
{
BinaryFormatter formatter = new BinaryFormatter();
string path = Application.persistentDataPath + "/playerData.dat";
FileStream stream = new FileStream(path, FileMode.Create);
formatter.Serialize(stream, data);
stream.Close();
}
public PlayerData LoadPlayerData()
{
string path = Application.persistentDataPath + "/playerData.dat";
if (File.Exists(path))
{
BinaryFormatter formatter = new BinaryFormatter();
FileStream stream = new FileStream(path, FileMode.Open);
PlayerData data = formatter.Deserialize(stream) as PlayerData;
stream.Close();
return data;
}
else
{
Debug.LogError("Save file not found in " + path);
return null;
}
}
}
바이너리 직렬화는 대용량 데이터를 빠르게 저장하고 불러올 때 유용해. 하지만 보안에 신경 써야 하고, 다른 플랫폼과의 호환성을 고려해야 해.
2.4 SQLite 데이터베이스 🗄️
SQLite는 경량 관계형 데이터베이스야. 복잡한 데이터 구조를 효율적으로 관리할 수 있어.
- 👍 장점: 복잡한 쿼리와 데이터 관계를 다룰 수 있고, 대용량 데이터 처리에 유리해.
- 👎 단점: 설정이 복잡하고, 간단한 데이터 저장에는 과도할 수 있어.
SQLite를 Unity에서 사용하려면 플러그인을 설치해야 해. 예를 들어, SQLite4Unity3d라는 플러그인을 사용할 수 있어. 사용 예제를 간단히 볼까?
using SQLite4Unity3d;
using UnityEngine;
public class PlayerData
{
[PrimaryKey, AutoIncrement]
public int Id { get; set; }
public string PlayerName { get; set; }
public int Level { get; set; }
public float Health { get; set; }
}
public class DatabaseManager
{
private SQLiteConnection _connection;
public DatabaseManager(string databasePath)
{
_connection = new SQLiteConnection(databasePath);
_connection.CreateTable<playerdata>();
}
public void SavePlayerData(PlayerData data)
{
_connection.InsertOrReplace(data);
}
public PlayerData LoadPlayerData(int id)
{
return _connection.Table<playerdata>().FirstOrDefault(x => x.Id == id);
}
}
// 사용 예시
public class GameManager : MonoBehaviour
{
private DatabaseManager _dbManager;
void Start()
{
string dbPath = Application.persistentDataPath + "/PlayerDatabase.db";
_dbManager = new DatabaseManager(dbPath);
// 데이터 저장
PlayerData newData = new PlayerData { PlayerName = "Unity마스터", Level = 10, Health = 100f };
_dbManager.SavePlayerData(newData);
// 데이터 불러오기
PlayerData loadedData = _dbManager.LoadPlayerData(1);
Debug.Log($"Loaded player: {loadedData.PlayerName}, Level: {loadedData.Level}");
}
}
</playerdata></playerdata>
SQLite는 복잡한 게임 데이터를 관리해야 할 때 강력한 도구가 될 수 있어. 하지만 간단한 게임이라면 JSON이나 바이너리 직렬화로도 충분할 거야.
팁: 재능넷에서 Unity 개발자를 찾을 때, 이런 다양한 데이터 저장 방식을 알고 있는 개발자를 선호한다고 해. 각 방식의 장단점을 이해하고 적절히 사용할 줄 아는 게 중요해!
자, 이제 Unity에서 사용할 수 있는 주요 데이터 저장 방식에 대해 알아봤어. 각각의 방식은 상황에 따라 장단점이 있으니, 프로젝트의 요구사항을 잘 파악하고 적절한 방식을 선택하는 게 중요해. 다음으로는 실제로 이런 방식들을 사용해서 세이브 시스템을 구현하는 방법을 자세히 알아볼 거야. 준비됐니? 🚀
3. Unity 모바일 게임 세이브 시스템 구현하기 🛠️
자, 이제 본격적으로 Unity에서 모바일 게임을 위한 세이브 시스템을 구현해볼 거야. 우리는 JSON 직렬화 방식을 사용할 건데, 이 방식이 가장 균형 잡힌 선택이라고 볼 수 있어. 복잡한 데이터도 저장할 수 있으면서 사용하기도 비교적 쉽거든. 그럼 시작해볼까? 🏁
3.1 저장할 데이터 정의하기 📝
먼저 우리가 저장하고 싶은 데이터를 정의해야 해. 예를 들어, 플레이어의 이름, 레벨, 경험치, 골드, 인벤토리 같은 정보를 저장한다고 생각해보자.
using System;
using System.Collections.Generic;
using UnityEngine;
[Serializable]
public class PlayerData
{
public string playerName;
public int level;
public float experience;
public int gold;
public List<inventoryitem> inventory;
}
[Serializable]
public class InventoryItem
{
public string itemName;
public int quantity;
}
</inventoryitem>
여기서 [Serializable] 속성은 이 클래스가 JSON으로 변환될 수 있다는 걸 Unity에게 알려주는 거야. 이렇게 하면 Unity의 JsonUtility가 이 클래스를 JSON으로 쉽게 변환할 수 있어.
3.2 세이브 시스템 매니저 만들기 🧠
이제 실제로 데이터를 저장하고 불러오는 역할을 할 매니저 클래스를 만들어보자. 이 클래스는 싱글톤 패턴을 사용해서 어디서든 쉽게 접근할 수 있게 만들 거야.
using UnityEngine;
using System.IO;
public class SaveSystemManager : MonoBehaviour
{
private static SaveSystemManager _instance;
public static SaveSystemManager Instance
{
get
{
if (_instance == null)
{
_instance = FindObjectOfType<savesystemmanager>();
if (_instance == null)
{
GameObject go = new GameObject("SaveSystemManager");
_instance = go.AddComponent<savesystemmanager>();
}
}
return _instance;
}
}
private void Awake()
{
if (_instance != null && _instance != this)
{
Destroy(this.gameObject);
}
else
{
_instance = this;
DontDestroyOnLoad(this.gameObject);
}
}
private string SavePath => $"{Application.persistentDataPath}/playerData.json";
public void SavePlayerData(PlayerData data)
{
string json = JsonUtility.ToJson(data);
File.WriteAllText(SavePath, json);
Debug.Log($"Data saved to {SavePath}");
}
public PlayerData LoadPlayerData()
{
if (File.Exists(SavePath))
{
string json = File.ReadAllText(SavePath);
PlayerData data = JsonUtility.FromJson<playerdata>(json);
Debug.Log($"Data loaded from {SavePath}");
return data;
}
else
{
Debug.LogWarning("Save file not found, creating a new player data.");
return new PlayerData();
}
}
public void DeletePlayerData()
{
if (File.Exists(SavePath))
{
File.Delete(SavePath);
Debug.Log($"Save file deleted from {SavePath}");
}
else
{
Debug.LogWarning("No save file found to delete.");
}
}
}
</playerdata></savesystemmanager></savesystemmanager>
이 SaveSystemManager는 싱글톤 패턴을 사용해서 게임 전체에서 하나의 인스턴스만 존재하도록 만들었어. 이렇게 하면 어느 스크립트에서든 SaveSystemManager.Instance
로 쉽게 접근할 수 있지.
주요 기능을 살펴볼까?
- 📥 SavePlayerData: PlayerData 객체를 JSON으로 변환해서 파일로 저장해.
- 📤 LoadPlayerData: 저장된 JSON 파일을 읽어서 PlayerData 객체로 변환해 줘.
- 🗑️ DeletePlayerData: 저장된 데이터 파일을 삭제해. 게임을 새로 시작하거나 할 때 유용할 거야.
주의: 실제 게임에서는 이 데이터를 암호화하는 것이 좋아. 그렇지 않으면 누군가 파일을 직접 수정해서 치트를 쓸 수 있거든. 나중에 암호화 방법도 알아볼 거야!
3.3 게임 매니저 만들기 🎮
이제 실제로 게임의 상태를 관리하고, 세이브 시스템을 사용할 게임 매니저를 만들어보자.
using UnityEngine;
public class GameManager : MonoBehaviour
{
private static GameManager _instance;
public static GameManager Instance
{
get
{
if (_instance == null)
{
_instance = FindObjectOfType<gamemanager>();
if (_instance == null)
{
GameObject go = new GameObject("GameManager");
_instance = go.AddComponent<gamemanager>();
}
}
return _instance;
}
}
private PlayerData _playerData;
private void Awake()
{
if (_instance != null && _instance != this)
{
Destroy(this.gameObject);
}
else
{
_instance = this;
DontDestroyOnLoad(this.gameObject);
}
LoadGame();
}
public void SaveGame()
{
SaveSystemManager.Instance.SavePlayerData(_playerData);
}
public void LoadGame()
{
_playerData = SaveSystemManager.Instance.LoadPlayerData();
}
public void NewGame()
{
_playerData = new PlayerData
{
playerName = "New Player",
level = 1,
experience = 0,
gold = 100,
inventory = new System.Collections.Generic.List<inventoryitem>()
};
SaveGame();
}
// 게임 데이터 접근/수정 메서드들
public string GetPlayerName() => _playerData.playerName;
public void SetPlayerName(string name)
{
_playerData.playerName = name;
SaveGame();
}
public int GetPlayerLevel() => _playerData.level;
public void LevelUp()
{
_playerData.level++;
SaveGame();
}
public float GetPlayerExperience() => _playerData.experience;
public void AddExperience(float exp)
{
_playerData.experience += exp;
SaveGame();
}
public int GetPlayerGold() => _playerData.gold;
public void AddGold(int amount)
{
_playerData.gold += amount;
SaveGame();
}
public void AddItemToInventory(string itemName, int quantity)
{
var existingItem = _playerData.inventory.Find(item => item.itemName == itemName);
if (existingItem != null)
{
existingItem.quantity += quantity;
}
else
{
_playerData.inventory.Add(new InventoryItem { itemName = itemName, quantity = quantity });
}
SaveGame();
}
}
</inventoryitem></gamemanager></gamemanager>
이 GameManager도 싱글톤 패턴을 사용했어. 이 클래스는 게임의 전반적인 상태를 관리하고, 플레이어 데이터를 수정할 때마다 자동으로 저장하는 역할을 해.
주요 기능을 살펴볼까?
- 🎮 NewGame: 새 게임을 시작할 때 호출돼. 초기 플레이어 데이터를 설정하고 저장해.
- 💾 SaveGame: 현재 플레이어 데이터를 저장해.
- 📂 LoadGame: 저장된 플레이어 데이터를 불러와.
- 🔄 기타 메서드들: 플레이어 이름 설정, 레벨 업, 경험치 추가, 골드 추가, 아이템 추가 등의 기능을 수행하고 자동으로 저장해.
팁: 재능넷에서 Unity 개발자를 구할 때, 이런 체계적인 구조를 만들 수 있는 개발자를 찾는 경우가 많아. 게임의 확장성과 유지보수를 위해서 정말 중요한 스킬이지!
3.4 UI 만들기 🖼️
이제 우리가 만든 세이브 시스템을 테스트할 수 있는 간단한 UI를 만들어보자. Unity의 UI 시스템을 사용해서 만들 거야.
먼저 Canvas를 만들고, 그 안에 다음과 같은 요소들을 추가해:
- 플레이어 이름을 표시할 Text
- 레벨을 표시할 Text
- 경험치를 표시할 Slider
- 골드를 표시할 Text
- '레벨 업' 버튼
- '골드 추가' 버튼
- '아이템 추가' 버튼
- '저장' 버튼
- '불러오기' 버튼
- '새 게임' 버튼
그리고 이 UI를 컨트롤할 스크립트를 만들어보자.
using UnityEngine;
using UnityEngine.UI;
public class UIManager : MonoBehaviour
{
public Text playerNameText;
public Text levelText;
public Slider experienceSlider;
public Text goldText;
public Button levelUpButton;
public Button addGoldButton;
public Button addItemButton;
public Button saveButton;
public Button loadButton;
public Button newGameButton;
private void Start()
{
UpdateUI();
levelUpButton.onClick.AddListener(OnLevelUpClicked);
addGoldButton.onClick.AddListener(OnAddGoldClicked);
addItemButton.onClick.AddListener(OnAddItemClicked);
saveButton.onClick.AddListener(OnSaveClicked);
loadButton.onClick.AddListener(OnLoadClicked);
newGameButton.onClick.AddListener(OnNewGameClicked);
}
private void UpdateUI()
{
playerNameText.text = $"Player: {GameManager.Instance.GetPlayerName()}";
levelText.text = $"Level: {GameManager.Instance.GetPlayerLevel()}";
experienceSlider.value = GameManager.Instance.GetPlayerExperience() / 100f; // 임의로 100을 최대 경험치로 설정
goldText.text = $"Gold: {GameManager.Instance.GetPlayerGold()}";
}
private void OnLevelUpClicked()
{ 네, 계속해서 UIManager 스크립트를 작성해 보겠습니다.
<pre><code>
private void OnLevelUpClicked()
{
GameManager.Instance.LevelUp();
UpdateUI();
}
private void OnAddGoldClicked()
{
GameManager.Instance.AddGold(10);
UpdateUI();
}
private void OnAddItemClicked()
{
GameManager.Instance.AddItemToInventory("Potion", 1);
Debug.Log("Added 1 Potion to inventory");
UpdateUI();
}
private void OnSaveClicked()
{
GameManager.Instance.SaveGame();
Debug.Log("Game Saved");
}
private void OnLoadClicked()
{
GameManager.Instance.LoadGame();
UpdateUI();
Debug.Log("Game Loaded");
}
private void OnNewGameClicked()
{
GameManager.Instance.NewGame();
UpdateUI();
Debug.Log("New Game Started");
}
}
이 UIManager 스크립트는 우리가 만든 UI 요소들과 GameManager를 연결해주는 역할을 해. 각 버튼이 클릭될 때마다 해당하는 GameManager의 메서드를 호출하고, UI를 업데이트하지.
3.5 테스트하기 🧪
이제 모든 준비가 끝났어! 게임을 실행해서 우리가 만든 세이브 시스템이 잘 작동하는지 테스트해보자.
- 새 게임 버튼을 눌러 새로운 게임을 시작해봐.
- 레벨 업 버튼을 몇 번 눌러 레벨을 올려봐.
- 골드 추가 버튼을 눌러 골드를 늘려봐.
- 아이템 추가 버튼을 눌러 인벤토리에 아이템을 추가해봐.
- 저장 버튼을 눌러 현재 상태를 저장해.
- 게임을 종료했다가 다시 실행해봐.
- 불러오기 버튼을 눌러 이전에 저장한 상태가 제대로 로드되는지 확인해봐.
팁: 실제 게임에서는 자동 저장 기능을 추가하는 것이 좋아. 예를 들어, 플레이어의 상태가 변경될 때마다 자동으로 저장하거나, 일정 시간마다 자동 저장을 하는 식이지. 이렇게 하면 플레이어가 실수로 저장을 잊어버리더라도 진행 상황을 잃지 않을 수 있어.
3.6 보안 강화하기 🔒
마지막으로, 우리가 만든 세이브 시스템의 보안을 강화해볼까? 현재는 JSON 파일을 그대로 저장하고 있어서, 누군가 파일을 직접 수정할 수 있어. 이를 방지하기 위해 간단한 암호화를 추가해보자.
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
using UnityEngine;
public class SaveSystemManager : MonoBehaviour
{
// ... (이전 코드는 그대로 유지)
private string EncryptionKey = "YourSecretKey123"; // 실제로는 더 복잡한 키를 사용해야 해
public void SavePlayerData(PlayerData data)
{
string json = JsonUtility.ToJson(data);
string encryptedJson = EncryptString(json);
File.WriteAllText(SavePath, encryptedJson);
Debug.Log($"Encrypted data saved to {SavePath}");
}
public PlayerData LoadPlayerData()
{
if (File.Exists(SavePath))
{
string encryptedJson = File.ReadAllText(SavePath);
string json = DecryptString(encryptedJson);
PlayerData data = JsonUtility.FromJson<playerdata>(json);
Debug.Log($"Decrypted data loaded from {SavePath}");
return data;
}
else
{
Debug.LogWarning("Save file not found, creating a new player data.");
return new PlayerData();
}
}
private string EncryptString(string text)
{
byte[] iv = new byte[16];
byte[] array;
using (Aes aes = Aes.Create())
{
aes.Key = Encoding.UTF8.GetBytes(EncryptionKey);
aes.IV = iv;
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
using (MemoryStream memoryStream = new MemoryStream())
{
using (CryptoStream cryptoStream = new CryptoStream((Stream)memoryStream, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter streamWriter = new StreamWriter((Stream)cryptoStream))
{
streamWriter.Write(text);
}
array = memoryStream.ToArray();
}
}
}
return Convert.ToBase64String(array);
}
private string DecryptString(string cipherText)
{
byte[] iv = new byte[16];
byte[] buffer = Convert.FromBase64String(cipherText);
using (Aes aes = Aes.Create())
{
aes.Key = Encoding.UTF8.GetBytes(EncryptionKey);
aes.IV = iv;
ICryptoTransform decryptor = aes.CreateDecryptor(aes.Key, aes.IV);
using (MemoryStream memoryStream = new MemoryStream(buffer))
{
using (CryptoStream cryptoStream = new CryptoStream((Stream)memoryStream, decryptor, CryptoStreamMode.Read))
{
using (StreamReader streamReader = new StreamReader((Stream)cryptoStream))
{
return streamReader.ReadToEnd();
}
}
}
}
}
}
</playerdata>
이렇게 하면 저장된 데이터가 암호화되어 일반 사용자가 쉽게 읽거나 수정할 수 없게 돼. 하지만 이 방법도 완벽한 보안을 제공하지는 않아. 실제 상용 게임에서는 더 복잡한 보안 방식을 사용하는 경우가 많지.
마무리 🎉
자, 이제 우리는 Unity로 모바일 게임을 위한 기본적인 세이브 시스템을 구현해봤어! 이 시스템은 다음과 같은 특징을 가지고 있지:
- 📊 구조화된 데이터 저장
- 💾 JSON을 이용한 직렬화
- 🔐 간단한 암호화
- 🎮 게임 매니저를 통한 중앙 집중식 데이터 관리
- 🖼️ UI를 통한 데이터 표시 및 조작
이 기본적인 구조를 바탕으로, 네트워크 동기화, 클라우드 저장, 더 복잡한 암호화 등을 추가해 나갈 수 있어. 게임의 규모와 요구사항에 따라 세이브 시스템을 계속 발전시켜 나가면 돼.
기억해! 세이브 시스템은 게임의 핵심 기능 중 하나야. 플레이어의 진행 상황을 안전하게 보관하고, 언제든 불러올 수 있게 해주는 중요한 역할을 하지. 재능넷에서도 이런 기능을 구현할 수 있는 개발자를 높이 평가한다고 해. 계속 연습하고 발전시켜 나가면, 넌 분명 훌륭한 게임 개발자가 될 수 있을 거야! 🚀
자, 이제 너만의 멋진 게임을 만들어볼 시간이야. 화이팅! 🎮✨