Unity 모바일 게임 세이브 시스템 구현 🎮💾
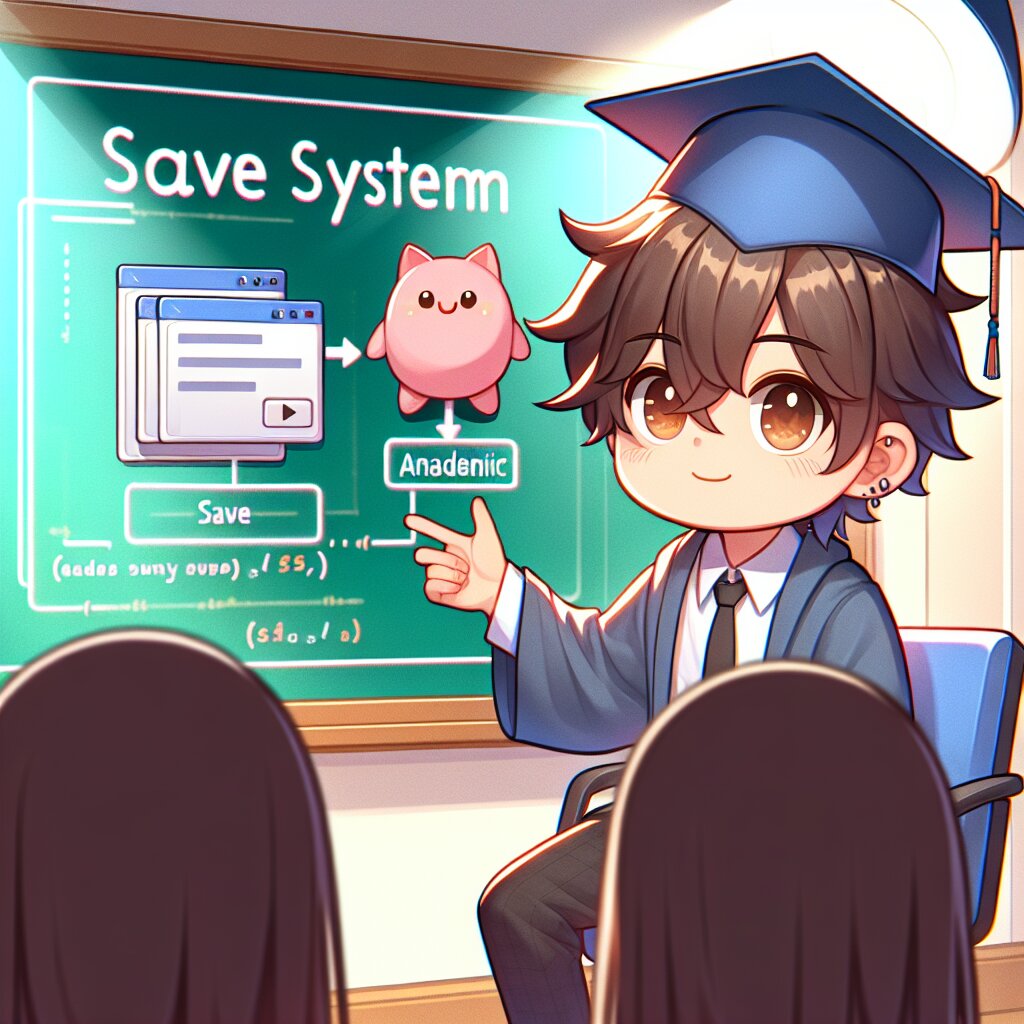
안녕, 게임 개발자 친구들! 오늘은 Unity로 모바일 게임을 만들 때 정말 중요한 주제인 '세이브 시스템 구현'에 대해 재미있게 얘기해볼 거야. 🚀 이 글을 읽고 나면 너도 게임 데이터를 저장하고 불러오는 마법사가 될 수 있을 거야! 😉
잠깐! 이 글은 재능넷(https://www.jaenung.net)의 '지식인의 숲' 메뉴에서 볼 수 있어. 재능넷은 다양한 재능을 거래하는 플랫폼이니, 게임 개발 관련 도움이 필요하다면 한 번 들러봐! 👀
자, 이제 본격적으로 시작해볼까? 🏁
1. 세이브 시스템이 왜 중요할까? 🤔
게임을 만들 때 가장 중요한 건 뭘까? 그래픽? 사운드? 아니면 재미있는 게임플레이? 다 맞는 말이지만, 플레이어의 진행 상황을 저장하는 것도 엄청 중요해! 왜 그런지 한번 생각해볼까?
- 📱 모바일 게임은 언제 어디서나 할 수 있어야 해
- 🔋 배터리가 떨어지거나 갑자기 앱이 종료되더라도 진행 상황이 날아가면 안 돼
- 🏆 플레이어의 업적이나 레벨 같은 중요한 정보를 기억해야 해
- 💰 인앱 구매 정보도 안전하게 저장해야 해
이런 이유들 때문에 세이브 시스템은 게임에서 절대 빠질 수 없는 핵심 기능이야. 재능넷에서도 게임 개발 관련 재능을 거래할 때 세이브 시스템 구현 능력은 정말 중요하게 여겨진다고 해. 그만큼 중요하다는 거지! 😎
주의사항: 세이브 시스템을 제대로 구현하지 않으면 플레이어들이 게임을 금방 지워버릴 수 있어. 그러니까 정말 신경 써서 만들어야 해!
자, 이제 Unity에서 어떻게 세이브 시스템을 구현하는지 하나씩 알아볼까? 🕵️♂️
2. Unity에서 사용할 수 있는 데이터 저장 방식 🗃️
Unity에서는 여러 가지 방법으로 데이터를 저장할 수 있어. 각각의 방식에는 장단점이 있으니, 상황에 맞게 선택해서 사용하면 돼. 어떤 방식들이 있는지 한번 살펴볼까?
2.1 PlayerPrefs 🍪
PlayerPrefs는 Unity에서 제공하는 가장 간단한 데이터 저장 방식이야. 마치 웹브라우저의 쿠키같은 거라고 생각하면 돼.
- 👍 장점: 사용하기 쉽고, 간단한 데이터를 빠르게 저장할 수 있어.
- 👎 단점: 보안이 취약하고, 복잡한 데이터 구조를 저장하기 어려워.
PlayerPrefs를 사용하는 간단한 예제를 볼까?
// 데이터 저장하기
PlayerPrefs.SetInt("Score", 100);
PlayerPrefs.SetString("PlayerName", "Unity마스터");
PlayerPrefs.Save();
// 데이터 불러오기
int score = PlayerPrefs.GetInt("Score", 0); // 기본값 0
string playerName = PlayerPrefs.GetString("PlayerName", "NoName"); // 기본값 "NoName"
PlayerPrefs는 간단한 설정이나 작은 양의 데이터를 저장할 때 유용해. 하지만 중요한 게임 데이터나 보안이 필요한 정보는 다른 방식으로 저장하는 게 좋아.
2.2 JSON 직렬화 📄
JSON(JavaScript Object Notation)은 데이터를 저장하고 전송하는 데 많이 사용되는 형식이야. Unity에서도 JSON을 이용해 데이터를 저장할 수 있어.
- 👍 장점: 복잡한 데이터 구조도 쉽게 저장할 수 있고, 가독성이 좋아.
- 👎 단점: PlayerPrefs보다는 조금 더 복잡하고, 암호화가 필요할 수 있어.
JSON을 사용한 데이터 저장 예제를 볼까?
using UnityEngine;
using System.IO;
[System.Serializable]
public class PlayerData
{
public string playerName;
public int level;
public float health;
}
public class SaveSystem : MonoBehaviour
{
public void SavePlayerData(PlayerData data)
{
string json = JsonUtility.ToJson(data);
File.WriteAllText(Application.persistentDataPath + "/playerData.json", json);
}
public PlayerData LoadPlayerData()
{
string path = Application.persistentDataPath + "/playerData.json";
if (File.Exists(path))
{
string json = File.ReadAllText(path);
return JsonUtility.FromJson<playerdata>(json);
}
else
{
Debug.LogError("Save file not found in " + path);
return null;
}
}
}
</playerdata>
JSON은 구조화된 데이터를 저장하기에 좋은 방식이야. 게임의 세이브 데이터처럼 여러 정보를 한꺼번에 저장해야 할 때 유용해.
2.3 바이너리 직렬화 💾
바이너리 직렬화는 데이터를 이진 형식으로 변환해서 저장하는 방식이야. 텍스트 기반의 JSON보다 더 효율적이고 빠르게 데이터를 처리할 수 있어.
- 👍 장점: 저장 공간을 적게 차지하고, 처리 속도가 빨라.
- 👎 단점: 사람이 직접 읽기 어렵고, 플랫폼 간 호환성 문제가 있을 수 있어.
바이너리 직렬화를 사용한 예제를 살펴볼까?
using UnityEngine;
using System.IO;
using System.Runtime.Serialization.Formatters.Binary;
[System.Serializable]
public class PlayerData
{
public string playerName;
public int level;
public float health;
}
public class SaveSystem : MonoBehaviour
{
public void SavePlayerData(PlayerData data)
{
BinaryFormatter formatter = new BinaryFormatter();
string path = Application.persistentDataPath + "/playerData.dat";
FileStream stream = new FileStream(path, FileMode.Create);
formatter.Serialize(stream, data);
stream.Close();
}
public PlayerData LoadPlayerData()
{
string path = Application.persistentDataPath + "/playerData.dat";
if (File.Exists(path))
{
BinaryFormatter formatter = new BinaryFormatter();
FileStream stream = new FileStream(path, FileMode.Open);
PlayerData data = formatter.Deserialize(stream) as PlayerData;
stream.Close();
return data;
}
else
{
Debug.LogError("Save file not found in " + path);
return null;
}
}
}
바이너리 직렬화는 대용량 데이터를 빠르게 저장하고 불러올 때 유용해. 하지만 보안에 신경 써야 하고, 다른 플랫폼과의 호환성을 고려해야 해.
2.4 SQLite 데이터베이스 🗄️
SQLite는 경량 관계형 데이터베이스야. 복잡한 데이터 구조를 효율적으로 관리할 수 있어.
- 👍 장점: 복잡한 쿼리와 데이터 관계를 다룰 수 있고, 대용량 데이터 처리에 유리해.
- 👎 단점: 설정이 복잡하고, 간단한 데이터 저장에는 과도할 수 있어.
SQLite를 Unity에서 사용하려면 플러그인을 설치해야 해. 예를 들어, SQLite4Unity3d라는 플러그인을 사용할 수 있어. 사용 예제를 간단히 볼까?
using SQLite4Unity3d;
using UnityEngine;
public class PlayerData
{
[PrimaryKey, AutoIncrement]
public int Id { get; set; }
public string PlayerName { get; set; }
public int Level { get; set; }
public float Health { get; set; }
}
public class DatabaseManager
{
private SQLiteConnection _connection;
public DatabaseManager(string databasePath)
{
_connection = new SQLiteConnection(databasePath);
_connection.CreateTable<playerdata>();
}
public void SavePlayerData(PlayerData data)
{
_connection.InsertOrReplace(data);
}
public PlayerData LoadPlayerData(int id)
{
return _connection.Table<playerdata>().FirstOrDefault(x => x.Id == id);
}
}
// 사용 예시
public class GameManager : MonoBehaviour
{
private DatabaseManager _dbManager;
void Start()
{
string dbPath = Application.persistentDataPath + "/PlayerDatabase.db";
_dbManager = new DatabaseManager(dbPath);
// 데이터 저장
PlayerData newData = new PlayerData { PlayerName = "Unity마스터", Level = 10, Health = 100f };
_dbManager.SavePlayerData(newData);
// 데이터 불러오기
PlayerData loadedData = _dbManager.LoadPlayerData(1);
Debug.Log($"Loaded player: {loadedData.PlayerName}, Level: {loadedData.Level}");
}
}
</playerdata></playerdata>
SQLite는 복잡한 게임 데이터를 관리해야 할 때 강력한 도구가 될 수 있어. 하지만 간단한 게임이라면 JSON이나 바이너리 직렬화로도 충분할 거야.
팁: 재능넷에서 Unity 개발자를 찾을 때, 이런 다양한 데이터 저장 방식을 알고 있는 개발자를 선호한다고 해. 각 방식의 장단점을 이해하고 적절히 사용할 줄 아는 게 중요해!
자, 이제 Unity에서 사용할 수 있는 주요 데이터 저장 방식에 대해 알아봤어. 각각의 방식은 상황에 따라 장단점이 있으니, 프로젝트의 요구사항을 잘 파악하고 적절한 방식을 선택하는 게 중요해. 다음으로는 실제로 이런 방식들을 사용해서 세이브 시스템을 구현하는 방법을 자세히 알아볼 거야. 준비됐니? 🚀